jQuery thumbnail scroller
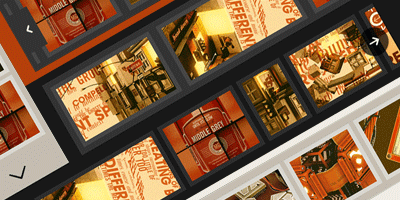
A thumbnail/image scroller that can be used as standalone or alongside lightboxes, gallery scripts etc. Features include: scrolling by cursor movement, buttons and/or touch, vertical and/or horizontal scrolling, customization via CSS and option parameters, methods for triggering events like scroll-to, update, destroy etc., user-defined callbacks functions and more.
Current version 2.0.3 (Changelog) – Version 1.x.x (no longer actively maintained)
How to use it
HTML
Get plugin files. Extract and upload jquery.mThumbnailScroller.min.js and jquery.mThumbnailScroller.css to your web server (more info)
Include jquery.mThumbnailScroller.css in the head tag your html document
<link rel="stylesheet" href="/path/to/jquery.mThumbnailScroller.css">
Include jQuery library (if your project doesn’t use it already) and jquery.mThumbnailScroller.min.js in your document’s head or body tag
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script> <script src="/path/to/jquery.mThumbnailScroller.min.js"></script>
Create the markup with your images, links, etc. which is basically an element (e.g. a div) holding an unordered list (ul)
<div id="my-thumbs-list"> <ul> <li><a href="image-1-link"><img src="/path/to/image-1-file" /></a></li> <li><a href="image-2-link"><img src="/path/to/image-2-file" /></a></li> <li><a href="image-3-link"><img src="/path/to/image-3-file" /></a></li> <li><a href="image-4-link"><img src="/path/to/image-4-file" /></a></li> <!-- and so on... --> </ul> </div>
CSS
Give your element (in the example above #my-thumbs-list) a width and/or height value and a CSS overflow value of auto or hidden. For example:
#my-thumbs-list{ overflow: auto; width: 800px; height: auto; }
If you’re creating a vertical scroller, you’ll need to set a height (or max-height) value
#my-thumbs-list{ overflow: auto; width: 300px; height: 500px; }
Initialization
Initialize via javascript
Create a script and call mThumbnailScroller function on your element selector along with the option parameters you want
<script> (function($){ $(window).load(function(){ $("#my-thumbs-list").mThumbnailScroller({ axis:"x" //change to "y" for vertical scroller }); }); })(jQuery); </script>
Initialize via HTML
If you prefer to initialize the plugin without a script, you can simply give your element the class mThumbnailScroller
and optionally set its orientation via the HTML data attribute data-mts-axis
("x"
for horizontal and "y"
for vertical). For example:
<div id="my-thumbs-list" class="mThumbnailScroller" data-mts-axis="x"> <ul> <!-- your content --> </ul> </div>
Basic configuration & option parameters
The 2 basic options are axis
which defines the scroller orientation and type
which defines how the user interacts with the scroller.
axis
The default scroller orientation is horizontal, so if you don’t set an axis
option value, it defaults to "x"
$("#my-thumbs-list").mThumbnailScroller({ axis:"x" });
To create a vertical scroller, set axis
option to "y"
$("#my-thumbs-list").mThumbnailScroller({ axis:"y" });
or use data-mts-axis="y"
if you’re initializing the plugin via HTML
type
You can set the type of scroller via javascript, for example:
$("#my-thumbs-list").mThumbnailScroller({ type:"hover-50" });
or using the HTML data attribute data-mts-type
, e.g.:
<div class="mThumbnailScroller" data-mts-type="hover-50">...</div>
There are 4 basic scroller types:
"hover-[number]"
(e.g."hover-33"
,"hover-80"
etc.)Scrolling content in linear mode by hovering the cursor over the scroller edges. The edges are defined by the type name, where [number] indicates the percentage of the scroller area in which scrolling is idle. For example, setting
"hover-50"
(default value) on a 600 pixels wide horizontal scroller, means that scrolling will be triggered only when the cursor is over the first 150 pixels from the left or right edge. And when cursor is over the 50% of the scroller width (300 pixels), scrolling will be idle or stopped.hover-precise
Scrolling content with animation easing (non-linear mode) by hovering the cursor over the scroller. The scrolling speed and direction is directly affected by the cursor movement and position within the scroller.
"click-[number]"
(e.g."click-50"
,"click-90"
etc.)Scrolling content by clicking buttons. The scrolling amount is defined by type name, where [number] indicates a percentage of the scroller area. For example, setting
"click-50"
on a 800 pixels wide horizontal scroller, means that each time you click the left or right arrow buttons, the content will scroll by 400 pixels (50% of 800px) to the left or right.click-thumb
Scrolling each image/thumbnail at a time by clicking buttons.
"hover-[number]"
and hover-precise
types become disabled on touch-enabled devices and replaced by content touch-swipe scrolling.
Configuration
You can configure your scroller(s) using the following option parameters when calling mThumbnailScroller function
Usage $(selector).mThumbnailScroller({ option: value });
setWidth: false
- Set the width of the scroller (overwrites CSS width), value in pixels (integer) or percentage (string).
setHeight: false
- Set the height of the scroller (overwrites CSS height), value in pixels (integer) or percentage (string).
setTop: 0
- Set the initial css top property of content, accepts string values (css top position).
Example:setTop: "-100px"
.
setLeft: 0
- Set the initial css left property of content, accepts string values (css left position).
Example:setLeft: "-100px"
.
type: "string"
- Scroller type defines the way users interact with the scroller.
Available values:"hover-[number]"
(e.g."hover-50"
)
Scrolling content in linear mode by hovering the cursor over the scroller edges. The edges are defined by the type name, where [number] indicates the percentage of the scroller area in which scrolling is idle. Scrolling accelerates proportionally according to cursor position: e.g. scrolling speed increases as the cursor moves towards the edges and decreases as it moves towards the center of the scroller area."hover-precise"
Scrolling content with animation easing (non-linear mode) by hovering the cursor over the scroller. The scrolling speed and direction is directly affected by the cursor movement and position within the scroller.click-[number]
(e.g."click-80"
)
Scrolling content by clicking buttons. The scrolling amount is defined by type name, where [number] indicates a percentage of the scroller area."click-thumb"
Scrolling each image/thumbnail at a time by clicking buttons. Each click will scroll the content by the width/height of the next non-visible adjusted image.
axis: "string"
- Define scroller axis (scrolling orientation).
Available values:"y"
,"x"
,"yx"
.axis: "y"
– vertical scrolleraxis: "x"
– horizontal scroller (default)axis: "yx"
– vertical and horizontal scroller (useful when panning images or switching axis on-the-fly)
speed: integer
- Set the scrolling speed (higher=faster). Default value is
15
.
contentTouchScroll: integer
- Enable or disable content touch-swipe scrolling for touch-enabled devices.
To completely disable, setcontentTouchScroll: false
. Integer values define the axis-specific minimum amount required for scrolling momentum (default value is25
).
markup:{ thumbnailsContainer: "string" }
- Set the element containing your thumbnails. By default, this is an unordered list (
ul
).
markup:{ thumbnailContainer: "string" }
- Set the element containing each thumbnail. By default this is a list-item (
li
).
markup:{ thumbnailElement: "string" }
- Set the actual thumbnail element. By default, this is an image tag (
img
).
advanced:{ autoExpandHorizontalScroll: boolean }
- Auto-expand content horizontally (for
"x"
or"yx"
axis).
If set totrue
(default), content will expand horizontally to accomodate any floated/inline-block elements (such asli
,a
,img
etc.).
advanced:{ updateOnContentResize: boolean }
- Update scroller(s) automatically on content, element or viewport resize. The value should be
true
(default) for fluid layouts/elements, adding/removing content dynamically, hiding/showing elements etc.
advanced:{ updateOnImageLoad: boolean }
- Update scroller(s) automatically each time an image inside the element is fully loaded. The value is
true
by default.
advanced:{ updateOnSelectorChange: "string" }
- Update scroller(s) automatically when the amount and size of specific selectors changes.
Useful when you need to update the scroller(s) automatically, each time a type of element is added, removed or changes its size.
For example, settingupdateOnSelectorChange: "ul li"
will update the scroller each time list-items inside the element are changed. Setting the value totrue
, will update the scroller each time any element is changed. To disable (default) set tofalse
.
theme: "string"
- Set a ready-to-use scroller theme (plugin’s CSS contains all themes).
callbacks:{ onInit: function(){} }
- A function/custom code to execute when the scroller has initialized (callbacks demo).
Example:
callbacks:{ onInit:function(){ console.log("scroller initialized"); } }
callbacks:{ onScrollStart: function(){} }
- A function/custom code to execute when scrolling starts (callbacks demo).
Example:
callbacks:{ onScrollStart:function(){ console.log("scroll started"); } }
callbacks:{ onScroll: function(){} }
- A function/custom code to execute when scrolling is completed (callbacks demo).
Example:
callbacks:{ onScroll:function(){ console.log("scroll completed"); } }
callbacks:{ onTotalScroll: function(){} }
- A function/custom code to execute when scrolling is completed and content is scrolled all the way to the end (bottom and/or right) (callbacks demo).
Example:
callbacks:{ onTotalScroll:function(){ console.log("Scrolled to 100%"); } }
callbacks:{ onTotalScrollBack: function(){} }
- A function/custom code to execute when scrolling is completed and content is scrolled back to the beginning (top and/or left) (callbacks demo).
Example:
callbacks:{ onTotalScrollBack:function(){ console.log("Scrolled back to 0%"); } }
callbacks:{ onTotalScrollOffset: integer }
- Set an offset for the onTotalScroll callback option.
For example, settingonTotalScrollOffset: 100
will trigger the onTotalScroll callback 100 pixels before the end of scrolling is reached.
callbacks:{ onTotalScrollBackOffset: integer }
- Set an offset for the onTotalScrollBack callback option.
For example, settingonTotalScrollBackOffset: 100
will trigger the onTotalScrollBack callback 100 pixels before the beginning of scrolling is reached.
callbacks:{ whileScrolling: function(){} }
- A function/custom code to execute while scrolling is active (callbacks demo).
Example:
callbacks:{ whileScrolling:function(){ console.log("scrolling..."); } }
callbacks:{ alwaysTriggerOffsets: boolean }
- Control the way onTotalScroll and onTotalScrollBack offsets execute.
By default, callback offsets will trigger repeatedly while content is scrolling within the offsets.
SetalwaysTriggerOffsets: false
when you need to trigger onTotalScroll and onTotalScrollBack callbacks only once.
live: boolean, "string"
- Enable or disable the creation of scroller(s) on all elements matching the current selector, now and in the future.
Setlive: true
when you need to add scroller(s) on elements that do not yet exist in the page. These could be elements added by other scripts or plugins after some action by the user.
If you need at any time to disable or enable the live option, setlive: "off"
and"on"
respectively.
You can also tell the script to disable live option after the first invocation by settinglive: "once"
.
liveSelector: "string"
- Set the matching set of elements to apply a scroller, now and in the future.
Plugin methods
Methods are ways to execute various scroller actions programmatically from within your script(s).
update
Usage $(selector).mThumbnailScroller("update");
Call the update method to manually update existing scrollers to accomodate new content or resized element(s). This method is by default called automatically by the script (via updateOnContentResize
option) when the element itself and/or its content size changes.
scrollTo
Usage $(selector).mThumbnailScroller("scrollTo", position, {options});
Call the scrollTo method to scroll content to the position parameter. Position parameter can be a string (e.g. "#element-id"
, "bottom"
, "left"
etc.), an integer indicating number of pixels (e.g. 100
), an array for y/x axis (e.g. [100,100]
), a js/jQuery object, a function etc. method demo
scrollTo position parameter
Position parameter can be:
"string"
- Element selector
For example, scroll to element with id “element-id”:
$(selector).mThumbnailScroller("scrollTo","#element-id");
- Special pre-defined position
For example, scroll to right:
$(selector).mThumbnailScroller("scrollTo","right");
Pre-defined position strings:
"bottom"
– scroll to bottom"top"
– scroll to top"right"
– scroll to right"left"
– scroll to left"first"
– scroll to the position of the first element within content"last"
– scroll to the position of the last element within content
- Number of pixels less/more: (e.g.
"-=100"
/"+=100"
)
For example, scroll by 100 pixels right:
$(selector).mThumbnailScroller("scrollTo","-=100");
- Element selector
integer
- Number of pixels (from left and/or top)
For example, scroll to 100 pixels:
$(selector).mThumbnailScroller("scrollTo",100);
- Number of pixels (from left and/or top)
[array]
- Different y/x position
For example, scroll to 100 pixels from top and 50 pixels from left:
$(selector).mThumbnailScroller("scrollTo",[100,50]);
- Different y/x position
object/function
- jQuery object
For example, scroll to element with id “element-id”:
$(selector).mThumbnailScroller("scrollTo",$("#element-id"));
- js object
For example, scroll to element with id “element-id”:
$(selector).mThumbnailScroller("scrollTo",document.getelementbyid("element-id"));
- function
For example, scroll to 100 pixels:
$(selector).mThumbnailScroller("scrollTo",function(){ return 100 });
- jQuery object
scrollTo method options
speed: integer
- Scrolling speed.
Example:
$(selector).mThumbnailScroller("scrollTo", "-=200", { speed: 30 });
duration: integer
- Scrolling animation duration, value in milliseconds.
Example:
$(selector).mThumbnailScroller("scrollTo", "right", { duration: 1000 });
easing: "string"
- Scrolling animation easing, values:
"easeInOut"
"easeOut"
"easeInOutSmooth"
"easeOutSmooth"
"easeInOutStrong"
"easeOutStrong"
"easeInOut"
"easeInOut"
Example:
$(selector).mThumbnailScroller("scrollTo", 300, { easing: "easeOutSmooth" });
callbacks: boolean
- Trigger user defined callbacks after scroll-to event is completed, values:
true
,false
.
Example:
$(selector).mThumbnailScroller("scrollTo", "top", { callbacks: false });
timeout: integer
- Method timeout (delay). The default timeout is 60 (milliseconds) in order to work with automatic scroller update functionality, value in milliseconds.
Example:
$(selector).mThumbnailScroller("scrollTo", "last", { timeout: 0 //no timeout });
stop
Usage $(selector).mThumbnailScroller("stop");
Stops running scrolling animations. Usefull when you wish to interrupt a previously scrollTo call.
disable
Usage $(selector).mThumbnailScroller("disable");
Calling disable method will temporarily disable the scroller(s). Disabled scrollers can be re-enabled by calling the update method.
To disable a scroller and reset its content position, call the method by setting its reset parameter to true
:
$(selector).mThumbnailScroller("disable", true);
destroy
Usage $(selector).mThumbnailScroller("destroy");
Calling destroy method will completely remove the scroller and return the element to its original state.
Scroller styling & themes
You can style your scroller(s) using jquery.mThumbnailScroller.css which contains the basic/default styling and few ready-to-use themes.
You can modify the default styling or an existing scroller theme directly, clone a theme and change it to your needs or overwrite the CSS in another stylesheet. If you wish to maintain compatibility with future plugin versions (that may include an updated version of jquery.mThumbnailScroller.css), I recommend using your own custom (or cloned) theme (creating your own custom theme), or overwriting the CSS. This way you can always upgrade jquery.mThumbnailScroller.css without having to redo your changes.
Themes
Plugin’s CSS includes a number of ready-to-use themes for quickly applying a basic styling to your scroller(s). To apply a theme, use theme
option parameter:
$(selector).mThumbnailScroller({ theme: "theme-name" });
or the HTML data attribute data-mts-theme
in your markup:
<div class="mThumbnailScroller" data-mts-theme="theme-name">...</div>
creating your own custom theme
User-defined callbacks
You can trigger your own js function(s) by using plugin’s callbacks option parameter (see all availbale callbacks in configuration section). Some examples:
$(selector).mThumbnailScroller({ callbacks:{ onScroll: function(){ /* do something */ } } });
$(selector).mThumbnailScroller({ callbacks:{ onTotalScroll: function(){ myFunction(); } } }); function myFunction(){ /* do something */ }
The plugin returns a number of values and objects that can be used inside the callbacks
this
– the scroller elementthis.mts.content
– the scrollable element containing the content (e.g. theul
element) as jquery objectthis.mts.top
– content’s top position (pixels)this.mts.left
– content’s left position (pixels)this.mts.topPct
– content vertical scrolling percentagethis.mts.leftPct
– content horizontal scrolling percentagethis.mts.direction
– content’s scrolling direction (returns"x"
or"y"
)
Plugin dependencies & requirements
- jQuery version 1.6.0 or higher
License
This work is released under the MIT License.
You are free to use, study, improve and modify it wherever and however you like.
https://opensource.org/licenses/MIT
Donating helps greatly in developing and updating free software and running this blog 🙂
Hi,
I am using this plugin in my wordpress theme and I would like the thumbnails to be bigger.
I have increased the size of the images in the .css file, but I also want the images to be responsive. So on a desktop, I want the images very large and on a mobile devise I want them to be smaller.
I have tried using %, but it doesn’t work, it only works when I give the images a fixed pixel value.
I have been using media queries to reduce the size of the images for each device. Is there a better way to do this?
Is there a setting to make the images responsive?
Thanks
Media queries is a good way to do this. If it works for your layout, you don’t have to change it.
Simple percentage won’t work with autoExpandHorizontalScroll option enabled (default) because scroller items are floated left and their container auto-expands to accommodate all of them horizontally. It could work if you disabled this option and set a fixed value for your items container (e.g.
ul
) but it wouldn’t make any difference for responsiveness.Perhaps you could set the images width in
vw
(viewport units) instead of percentage. For example,width: 15vw;
will set an element’s width 15% of the viewport (window) width.It would be more complete if there was and the code for displaying the zoom image when clicking on a thumbnail.
Thanks for the suggestion Tasso.
The plugin is basically a scroller and implementing an image viewer (e.g. a lightbox) within the script is probably out of its scope. The plugin can be used to scroll thumbnails, large images, divs etc. so adding an image viewer would only apply on thumbnails.
You can easily implement any kind of lightbox/modal plugin with the scroller so you have more freedom on which one to use. I’ll probably create a tutorial and maybe some extension for this at some point 🙂
The buttons are not appearing
Send me your link or some test page, otherwise I can’t help.
Hi,
How do I change the direction using the RTL option?
If your document is right-to-left then the scroller changes automatically. To set document direction to rtl use CSS direction property (https://developer.mozilla.org/en/docs/Web/CSS/direction) or set the dir attribute in html tag (e.g.
<html dir="rtl">
)Hi!
This is an excellent plugin. Very impressive range of examples.
For the scroller that has left and right buttons (simple_example.html and scrollTo_demo.html ), how do add more rows of scrolling thumbnails to the same page? I’ve tried adding
<!-- demo content --> <a href="#" rel="nofollow"></a> <a href="#" rel="nofollow"></a> <a href="#" rel="nofollow"></a> <a href="#" rel="nofollow"></a> <a href="#" rel="nofollow"></a> <a href="#" rel="nofollow"></a> <a href="#" rel="nofollow"></a> <a href="#" rel="nofollow"></a> <a href="#" rel="nofollow"></a> <a href="#" rel="nofollow"></a> <a href="#" rel="nofollow"></a> <a href="#" rel="nofollow"></a> <a href="#" rel="nofollow"></a> <a href="#" rel="nofollow"></a> <a href="#" rel="nofollow"></a> <!-- -//- -->
Below the first scroller’s closing but it doesn’t work – shows up as a stacked bulleted list of thumbnails.
Is there something I need to change in the html and js files?
Thanks,
Helen
<!-- demo content --> <div id="content-1" class="content"> <ul> <li><a href="#"><img src="thumbnails/img1.jpg" /></a></li> <li><a href="#"><img src="thumbnails/img2.jpg" /></a></li> <li><a href="#"><img src="thumbnails/img3.jpg" /></a></li> <li><a href="#"><img src="thumbnails/img4.jpg" /></a></li> <li><a href="#"><img src="thumbnails/img5.jpg" /></a></li> <li><a href="#"><img src="thumbnails/img6.jpg" /></a></li> <li><a href="#"><img src="thumbnails/img7.jpg" /></a></li> <li><a href="#"><img src="thumbnails/img8.jpg" /></a></li> <li><a href="#"><img src="thumbnails/img9.jpg" /></a></li> <li><a href="#"><img src="thumbnails/img10.jpg" /></a></li> <li><a href="#"><img src="thumbnails/img11.jpg" /></a></li> <li><a href="#"><img src="thumbnails/img12.jpg" /></a></li> <li><a href="#"><img src="thumbnails/img13.jpg" /></a></li> <li><a href="#"><img src="thumbnails/img14.jpg" /></a></li> <li><a href="#"><img src="thumbnails/img15.jpg" /></a></li> </ul> </div> <!-- -//- -->
In simple_example.html clone #content-1 element markup and CSS to an element with id #content-0 and change the function call to:
$("#content-0, #content-1").mThumbnailScroller({ type:"click-50", theme:"buttons-out" });
Thank-you! That solved the issue
Awesome plugin Malihu, thank you very much for developing and sharing it. It has a huge degree of flexibility! I made a donation as a token of my appreciation for the countless hours of programming that your plugin saved me.
If I may, I would suggest only one enhancement, and that would be to give the option to allow the scroller to scroll automatically until the user hovers on it. Once it does, the control scheme would revert back to the one currently implemented (clicks on scroll buttons or mouse hover). Thanks!
Thanks a lot for the donation, I really appreciate the support 🙂
Also thanks for the suggestion! Have you seen the auto_scroll_example.html (demo) in plugin archive? It does what you describe via the callback options (fn call).
I am successfully using your scroller (wonderful), but I would like to add the auto-scroll capability… I can’t figure out how to add your example code into this script to make it work… This is what I have so far:
jQuery(document).ready(function()
{
$(“.vcarousel”).mThumbnailScroller({
theme:”hover-classic”,
axis:”y”
});
});
Where would I add the callbacks section from your sample code?
You probably need to change right/left to bottom/top. The following should work…
(function($){ $(window).load(function(){ var loopDuration=6000; $(".vcarousel").mThumbnailScroller({ theme:"hover-classic", axis:"y", callbacks:{ onInit:function(){ $(".vcarousel").mThumbnailScroller("scrollTo","bottom",{ duration:loopDuration/2,easing:"easeInOutSmooth" }); }, onTotalScroll:function(){ if($(this).data("mTS").trigger==="external"){ $(".vcarousel").mThumbnailScroller("scrollTo","top",{ duration:loopDuration/2,easing:"easeInOutSmooth" }); } } } }); }); })(jQuery);
It’s better to run the code on window load (instead of document ready) so all images are loaded before auto-scrolling starts.
Thank you very much for your help… Especially the “$(window).load(function()” part… That makes all the difference!
No problem 🙂
You need the window load wrapper because auto-scroll code has to run after all images are loaded.
This is a great plugin, the hover-precise is exactly what I want. However, because our site is hosted on shopify, and I could only find one thread on Shopify which has halt for posting any comments anymore, I have some difficulty to display all products in a horizontal scroll bar on the homepage, wondering if can ask some help.
I got the full list of products and images, but just cannot initiate the calling function. Is there a post/place address the shopify intigration issues?
The scroll bar we want to place is on the home page of http://www.gobiorganic.co.uk.
password:gobiorganic
Many thanks for your help in advance,
Frances
Hi, this is a great scroller. I will try to look back at the comments, but if you can answer, that would be great. while testing this scroller inside android devices and apple device the result is different.Inside Apple devices and their browser the smoothness of slider is great but if check inside android native browser or chrome browser it doesn’t work fine.the smoothness is not comparable with ios devices result.
what should i do too apply same smoothness for all devices.
Hope you will be reply soon.
If I want the scroller to display all the images in a specific folder, instead of listing each image separately, is that possible? How would I do that?
This should be done server-side (e.g. with PHP).
There are ways to do it via js but I’d suggest doing it with PHP.
Okay, thank you. I don’t see how this interacts with your thumbnail scroller though… to make the scroller see and load the files. Also, this is on a Java site on Tomcat… Any hints?
The server-side script (e.g. PHP) should read the dir and generate the markup based on the images it finds. The complete HTML (ul, li, img tags etc.) will be fully created by the server-side script, so the front-end scripts like the thumbnail scroller will work with the generated markup (the same way as if you wrote it by hand). The point is that instead of creating the HTML manually, the server-side script will do it for you.
I can’t help with Java/Tomcat as I don’t have any experience with it.
Hi there,
I’m having a problem with the plugin .. what happenings is that I’m using it on a grid .. it’s looking smth like this :
The width of the mTs_1_container is not calculated properly and resize is not at a 100% width .. the ” mThumbnailScroller” div is 100% though ..
Initialize:
(function($) {
$(window).load(function() {
$(“.grid-content-wrapper”).mThumbnailScroller({
type: “click-25”
});
$(“#info a”).click(function(e) {
e.preventDefault();
var $this = $(this),
el = $this.attr(“rel”),
to = $this.attr(“href”).split(“#”)[1];
if (to === “center-white”) {
to = $(“#white”).position().left + ($(“#white”).width() / 2) – ($(el).find(“.mTSWrapper”).width() / 2);
} else if (to === “yellow”) {
to = $(“#yellow”);
} else if (to === “by-100”) {
to = “-=100”;
}
$(el).mThumbnailScroller(“scrollTo”, to);
});
});
})(jQuery);
Tried the setWidth options with no succes .. if I change the width auto from here a.advanced.autoExpandHorizontalScroll && “y” !== a.axis && i.css({position: “absolute”, width: “auto”})
looks ok when the page loads .. only problem is that the scroll is not working properly anymore.
Any help please ?
Thank you.
div class=”new-grid-content-wrapper mThumbnailScroller _mTS_1 mTS-none mTS_no_scroll”
div id=”mTS_1″ class=”mTSWrapper mTS_horizontal”
div id=”mTS_1_container” class=”mTSAutoContainer mTSContainer” style=”position: relative; top: 0px; left: 0px; width: 1463px;”
table width=”100%”
I cannot paste html content ?
The problem would lay in the calculation of the autoContainer .. with is too small .. width: 1463px;
grid-content-wrapper i mean above .. the selector is ok …
To paste code use the code tag (see info below comment field).
Hi, this is a great scroller. I will try to look back at the comments, but if you can answer, that would be great. I was wondering if I can change the amount the scroller moves in distance when clicking on the right and left arrows. It seems to move about half way, but I would like it to jump to the next thumbnails. Where can I change that? Thanks much!
Hello,
To scroll by each thumbnail at a time, you set the type option parameter value to
"click-thumb"
, for example:$("#my-thumbs-list").mThumbnailScroller({ type:"click-thumb" });
Hi malihu, thanks so much for responding. I realize I was a little unclear above. What I want is the scroller to move the full width of the scroller. So for instance, I have a width set of 624px with 6 images appearing. Currently, when I click the arrow, it moves about 3 images forward, rather than 6. Would I need to adjust “click-[number]”?
Yes. The [number] indicates the scroller area percentage, so you probably need to set it to:
"click-100"
So I found this code at the end of the page:
(function($){
$(window).load(function(){
$(“#content-1″).mThumbnailScroller({
type:”click-50″,
theme:”buttons-out”
});
});
})(jQuery);
I tried changing it to “click-100” but no change.
It should work.
Are you working on the demo or?
Thanks for your help. It does work on the demo, so it must be conflicting with something on my side. I’ll figure it out 🙂
I think that you might be initializing the scroller via HTML, which means that the script won’t work.
Do you apply the scroller by adding the
mThumbnailScroller
class to your element?If yes, you don’t need the script to set the type option. You can set it via the HTML attribute
data-mts-type
. For example:<div class="mThumbnailScroller" data-mts-type="click-100">...</div>
The script:
$(selector).mThumbnailScroller({ type:"click-100" });
will work when initializing the scroller via javascript (when your element does not have the
mThumbnailScroller
class).Hope this helps 🙂
I am using your plugin it is awesome . It is working properly except one problem. Here we are using widget which is reused on different pages . I am using this widget on three pages . In First page i am using this for text scrolling . Second page for image scrolling . In third page i dont want to use it so i deleted it using “destroy” method . I am calling this destroy method while loading the third page . But my problem is in third page i am loading one spinner image . Afer calling destroy method that spinner image is not getting loaded . Only after refreshing the page it is getting loaded . And also sometimes if i am going back to the second page the images are not getting loaded . So i am refreshing the page it is getting loaded . And the same is happening for first page there text is not getting loaded . So what could be the cause of this problem. But i am sure it is happening only after calling destroy method. Can you please look at in this issue and provide me any solution ? Any method should i call after calling destroy method ?
I can’t really say unless I see your code and what exactly happens… Can you send me a link?
applyThumbnailScroll : function(){
var thisObj = this;
var currentElement = $(“.cls_skQuiz_sk_show”, this.el);
var isRangeSelector = $(currentElement).find(“.cls_skQuiz_rangeSelector”).length > 0;
var isTxtQuestion = $(currentElement).find(“.cls_skQuizText”).length > 0;
var thumbnailElem = “img”;
if(isTxtQuestion){
if( thisObj.properties[“textScroll”] != “Horizontal scroll”){
return ;
}
thumbnailElem = “.cls_skQuiz_optContent”;
} else {
if( thisObj.properties[“imageScroll”] != “Horizontal scroll”){
return ;
}
}
if(isRangeSelector){
$(“.cls_skQuiz_ans”).mThumbnailScroller(“destroy”);
$(“.cls_skQuiz_ans”).mThumbnailScroller(“update”);
return;
}
if(!isRangeSelector){
$(“.cls_skQuiz_ans_scroll”).mThumbnailScroller({
axis: “x”, //change to “y” for vertical scroller
type:”click-50″,
timeout: 0,
markup: {
thumbnailsContainer: “div”,
thumbnailContainer: “div”,
buttonsHTML:{
left:””, //plain text
right:”” //HTML right-arrow code
}
}
});
}
},
That above code i am using .Can you find any bug ?
Hai sorry it was my mistake . I have changed the selector so the problem has been solved .
is there a way to put some content (i.e a with something on each thumb ?
See the reply to a similar question below:
http://manos.malihu.gr/jquery-thumbnail-scroller/comment-page-13/#comment-19764
Dear Malihu,
thank you for your amazing plugin. I’m just curious – is it possible to have two types of scrolling activated at same time? I can not figure it out myself so I need your help.
I need to have “hover type” and “buttons” at same time. Click my website link, there is screen (really!¨) of what I need. If you need donation for this, just tell me.
Sincerely,
Rob
You cannot have 2 types at the same time, but you might able to do it manually with 2 extra buttons and the scrollTo method.
I made a quick example using the scrollTo method on 2 extra buttons (view source to see/get the code):
http://manos.malihu.gr/repository/jquery-thumbnail-scroller/demo/examples/hover-with-buttons-test.html
I’m also posting some demo code:
HTML
<div id="content-1"> <!-- extra next/previous buttons --> <p class="next-prev-btns"> <a href="#previous">‹</a> <a href="#next">›</a> </p> <ul> <li><a href="#"><img src="thumbnails/img3.jpg" /></a></li> <li><a href="#"><img src="thumbnails/img7.jpg" /></a></li> <li><a href="#"><img src="thumbnails/img11.jpg" /></a></li> <!-- and so on... --> </ul> </div>
JS
(function($){ $(window).load(function(){ var el=$("#content-1"), toggleClass="inactive"; el.mThumbnailScroller({ type:"hover-50", callbacks:{ onInit:function(){ $(".next-prev-btns a[href='#previous']").addClass(toggleClass); }, onScroll:function(){ $(".next-prev-btns > a").removeClass(toggleClass); }, onTotalScroll:function(){ $(".next-prev-btns a[href='#next']").addClass(toggleClass); }, onTotalScrollBack:function(){ $(".next-prev-btns a[href='#previous']").addClass(toggleClass); } } }); $(".next-prev-btns > a").click(function(e){ e.preventDefault(); var val=152, //amount of pixels to scroll-to each time buttons are clicked (e.g. 152, el.width() etc.) dir=$(this).attr("href")==="#previous" ? "+=" : "-="; if(!el.data("mTS").tweenRunning && !$(this).hasClass(toggleClass)){ //triggered only when scroller is idle and buttons is active el.mThumbnailScroller("scrollTo",dir+val,{duration:500}); } }); }); })(jQuery);
Is there a limit to the number of galleries per page? I got up to seven – but eight won’t work?
No, there’s no limit. Maybe some CSS rule is off on your 8th scroller?
Congratulations for your work! It is really good!
Please, see if you can help me. I am trying to use the type: “click-thumb” and use my own buttons however it is not working. I always get two black squares and not my png files.
Below is my code..
$(“#my-thumbs-list”).mThumbnailScroller({
axis:”x”,
type: “click-thumb”,
markup:{
buttonsHTML:{
left:””,
right:””
}
}
});
thanks in advance!
Not sure in my previous post why the left and right were in blank. There I added the img tag:
Hi there,
Thanks for your great plugin! I am using this on one of my client website and it work fine, but only one issue. So my client want to have the left / right mouse cursor trigger points in to the center more. Looking at the document, I tried to use “type” option, so my code as below:
$('.em-image-scroller').mThumbnailScroller({ speed:50, type:"hover-90" });
But it does not change anything.. the left/right area still far away from the center.
Currently I am using
type: "hover-precise"
to have a better left/right area, but really wish to have “hover-90” work.Could you please look into this issue.
Many thanks again for your great plugin!
i want a thumbnail with a description to the right .i am not able to figure it out
hi that is a nice plugin . is it possible to scroll thumbnail automatically and constantly like a loop?
Hi,
Thank you for hte wonderful plugin. When I use the following configuration, the first click on the arrow scrolls the scroller all the way to the last slide. Then scrolling backwards works as you would expect, one slide at a time. Any ideas? Thanks! Charles
—
$("#content-1").mThumbnailScroller({ type:"click-thumb", theme:"buttons-out" });
Hi,
How and where can I set the thumbnails to be centered in the scroller ?
For example, if my scroller, which take the full width, has only 2 or 3 thumbnails, I want them to be in center instead of left.
Regards.
I am wondering if this plugin can be used for Div instead of lists.
If possible can you guide how to use it ?
Yes, it can basically scroll anything.
See the markup options which you can use to set your own element selectors.
For example:
markup:{ thumbnailsContainer: "#my-id", //instead of "ul" thumbnailContainer: ".my-class" //instead of "li" }
Hi,
I want to build a web site that is responsive and looks something like http://tympanus.net/Tutorials/AnimatedPortfolioGallery/. I want the thumbnail scroller on bottom running horizontally (so it is responsive). When a thumbnail is selected, I need to use JQuery to do some easing of the related information like they do in AnimatedPortfolioGallery.
I downloaded your GREAT software and tried to figure out your example ‘simple-example.html’ and ‘call-backsdemo.html’ ,but I could not figure out how to get from your example to what I want the web page to do.
Anyway, do you have an example that is something like what I am trying to do ?
Thank you in advance!
Hello,
The thumbnail scroller should work independently from the rest of the gallery. You could add a click event on the thumbnails to do whatever you like. The plugin only handles the scrolling of the images.
Thank you for the information. I spent 30 years using COBOL so this is very different for me. Do you know of any sites that I can look at to see how the click events were used? Any examples would be most appreciated!
Thank you in advance.
I’m refering to jQuery (or javascript) click events. You’ll need basic knowledge in HTML and CSS to understand and work with js.
jQuery documentation: https://api.jquery.com/
jQuery click event: https://api.jquery.com/click/
Hi. It is great job. I use it, but after that need horizontal scroller.
Is there a way to make it responsive? I noticed that the demo is not responsive. Mind you, I may have missed something … I am not an expert … therefore need help.
Nick
The plugin script does not mess with the responsiveness. You can do pretty much anything you want with it via CSS, JS or both. You can even change axis like in the css_media_queries_demo.html.
If you need help with a specific layout that doesn’t seem to work, let me know.
awesome and really creative ui component, i want to use this both on touch and with mouse, i am having an issue whenever i use touch, the mouse hovering does stops working… I am sorry if this is a repetitive question, i really appreciate you taking the time out for answering so many questions!
The scroller has a specific scrolling behavior regarding touch. It basically works the same as any overflowed content which is by touch-swipe. What do you mean by “hovering stops working”? Can you provide more details?
hello ive made the update but the the right button its still there. can you help me?
Hi this is a great plugin Thank YOU!
I found the comment here where someone asked how to hide the scroll button when buttons are “out” and there is no content to scroll to the right, per se, within the container upon initial load.
I have updated the css to have line 130 in css to:
.mTSButton.mTS-hidden,
.mThumbnailScroller.mTS_no_scroll .mTSButton{
I am initializing it using the theme:
theme:”buttons-in”
Yet, I still get a button to scroll right when there is no more content to scroll to (all list items are visible). Yet on menus where I have more items and I can scroll all the way to the right/end, the right arrow ‘does’ hide itself knowing there is nothing more to show.
Wondering if my theme setting is not allowing the button to hide itself with this css change? What else can I look into? Any help would be appreciated.
Hello,
Normally, this shouldn’t happen. Are you using the latest version (2.0.2)? If not, just get version 2.0.2 which has this fix:
https://github.com/malihu/thumbnail-scroller/releases
Let me know if you still have this issue
hello ive made the update but the the right button its still there. can you help me?
I figured out the multiple galleries on the same page.
But, if you could tell which value/property changes the spacing of the thumbnails, that would be great. I can’t figure that out.
Nick
The CSS property is the margin of list-items. In demo.html, this is defined on line 35 (.content li):
https://github.com/malihu/thumbnail-scroller/blob/master/examples/demo.html#L35
In addition, each list item gets the class “mTSThumbContainer” so you could target this class if you want, e.g.
.content .mTSThumbContainer{ margin: 4px 0; }
I love this and thank you for making it available for the rest of us.
I have 2 questions that you may have already answered.
How can I have 2 different galleries on the same page?
How do I change the spacing (padding) of the thumbnails so they are not so far apart.
Nick
I’d like to capture mouse events while the div scrolls, so I can say which item the mouse is over while the user is scrolling. I’d like to know exactly which item the mouse is over.
How can I do this? jQuery .hover(), .mouseenter() etc only fire on mouse move, when the slideshow scroller changes position just on hovering.
I think that you’ll need a timer for this (e.g. a setInterval function) to constantly detect the values without relying on mouse/scroller movement. Maybe you could use plugin’s whileScrolling callback to call your own functions instead of creating a new timer.
Of course, depending on what you want to do when cursor is over an item, it might have an impact on overall performance.