jQuery thumbnail scroller
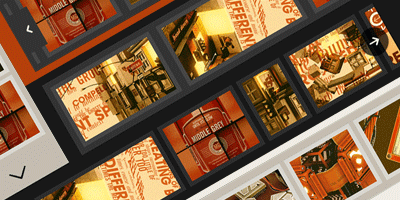
A thumbnail/image scroller that can be used as standalone or alongside lightboxes, gallery scripts etc. Features include: scrolling by cursor movement, buttons and/or touch, vertical and/or horizontal scrolling, customization via CSS and option parameters, methods for triggering events like scroll-to, update, destroy etc., user-defined callbacks functions and more.
Current version 2.0.3 (Changelog) – Version 1.x.x (no longer actively maintained)
How to use it
HTML
Get plugin files. Extract and upload jquery.mThumbnailScroller.min.js and jquery.mThumbnailScroller.css to your web server (more info)
Include jquery.mThumbnailScroller.css in the head tag your html document
<link rel="stylesheet" href="/path/to/jquery.mThumbnailScroller.css">
Include jQuery library (if your project doesn’t use it already) and jquery.mThumbnailScroller.min.js in your document’s head or body tag
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script> <script src="/path/to/jquery.mThumbnailScroller.min.js"></script>
Create the markup with your images, links, etc. which is basically an element (e.g. a div) holding an unordered list (ul)
<div id="my-thumbs-list"> <ul> <li><a href="image-1-link"><img src="/path/to/image-1-file" /></a></li> <li><a href="image-2-link"><img src="/path/to/image-2-file" /></a></li> <li><a href="image-3-link"><img src="/path/to/image-3-file" /></a></li> <li><a href="image-4-link"><img src="/path/to/image-4-file" /></a></li> <!-- and so on... --> </ul> </div>
CSS
Give your element (in the example above #my-thumbs-list) a width and/or height value and a CSS overflow value of auto or hidden. For example:
#my-thumbs-list{ overflow: auto; width: 800px; height: auto; }
If you’re creating a vertical scroller, you’ll need to set a height (or max-height) value
#my-thumbs-list{ overflow: auto; width: 300px; height: 500px; }
Initialization
Initialize via javascript
Create a script and call mThumbnailScroller function on your element selector along with the option parameters you want
<script> (function($){ $(window).load(function(){ $("#my-thumbs-list").mThumbnailScroller({ axis:"x" //change to "y" for vertical scroller }); }); })(jQuery); </script>
Initialize via HTML
If you prefer to initialize the plugin without a script, you can simply give your element the class mThumbnailScroller
and optionally set its orientation via the HTML data attribute data-mts-axis
("x"
for horizontal and "y"
for vertical). For example:
<div id="my-thumbs-list" class="mThumbnailScroller" data-mts-axis="x"> <ul> <!-- your content --> </ul> </div>
Basic configuration & option parameters
The 2 basic options are axis
which defines the scroller orientation and type
which defines how the user interacts with the scroller.
axis
The default scroller orientation is horizontal, so if you don’t set an axis
option value, it defaults to "x"
$("#my-thumbs-list").mThumbnailScroller({ axis:"x" });
To create a vertical scroller, set axis
option to "y"
$("#my-thumbs-list").mThumbnailScroller({ axis:"y" });
or use data-mts-axis="y"
if you’re initializing the plugin via HTML
type
You can set the type of scroller via javascript, for example:
$("#my-thumbs-list").mThumbnailScroller({ type:"hover-50" });
or using the HTML data attribute data-mts-type
, e.g.:
<div class="mThumbnailScroller" data-mts-type="hover-50">...</div>
There are 4 basic scroller types:
"hover-[number]"
(e.g."hover-33"
,"hover-80"
etc.)Scrolling content in linear mode by hovering the cursor over the scroller edges. The edges are defined by the type name, where [number] indicates the percentage of the scroller area in which scrolling is idle. For example, setting
"hover-50"
(default value) on a 600 pixels wide horizontal scroller, means that scrolling will be triggered only when the cursor is over the first 150 pixels from the left or right edge. And when cursor is over the 50% of the scroller width (300 pixels), scrolling will be idle or stopped.hover-precise
Scrolling content with animation easing (non-linear mode) by hovering the cursor over the scroller. The scrolling speed and direction is directly affected by the cursor movement and position within the scroller.
"click-[number]"
(e.g."click-50"
,"click-90"
etc.)Scrolling content by clicking buttons. The scrolling amount is defined by type name, where [number] indicates a percentage of the scroller area. For example, setting
"click-50"
on a 800 pixels wide horizontal scroller, means that each time you click the left or right arrow buttons, the content will scroll by 400 pixels (50% of 800px) to the left or right.click-thumb
Scrolling each image/thumbnail at a time by clicking buttons.
"hover-[number]"
and hover-precise
types become disabled on touch-enabled devices and replaced by content touch-swipe scrolling.
Configuration
You can configure your scroller(s) using the following option parameters when calling mThumbnailScroller function
Usage $(selector).mThumbnailScroller({ option: value });
setWidth: false
- Set the width of the scroller (overwrites CSS width), value in pixels (integer) or percentage (string).
setHeight: false
- Set the height of the scroller (overwrites CSS height), value in pixels (integer) or percentage (string).
setTop: 0
- Set the initial css top property of content, accepts string values (css top position).
Example:setTop: "-100px"
.
setLeft: 0
- Set the initial css left property of content, accepts string values (css left position).
Example:setLeft: "-100px"
.
type: "string"
- Scroller type defines the way users interact with the scroller.
Available values:"hover-[number]"
(e.g."hover-50"
)
Scrolling content in linear mode by hovering the cursor over the scroller edges. The edges are defined by the type name, where [number] indicates the percentage of the scroller area in which scrolling is idle. Scrolling accelerates proportionally according to cursor position: e.g. scrolling speed increases as the cursor moves towards the edges and decreases as it moves towards the center of the scroller area."hover-precise"
Scrolling content with animation easing (non-linear mode) by hovering the cursor over the scroller. The scrolling speed and direction is directly affected by the cursor movement and position within the scroller.click-[number]
(e.g."click-80"
)
Scrolling content by clicking buttons. The scrolling amount is defined by type name, where [number] indicates a percentage of the scroller area."click-thumb"
Scrolling each image/thumbnail at a time by clicking buttons. Each click will scroll the content by the width/height of the next non-visible adjusted image.
axis: "string"
- Define scroller axis (scrolling orientation).
Available values:"y"
,"x"
,"yx"
.axis: "y"
– vertical scrolleraxis: "x"
– horizontal scroller (default)axis: "yx"
– vertical and horizontal scroller (useful when panning images or switching axis on-the-fly)
speed: integer
- Set the scrolling speed (higher=faster). Default value is
15
.
contentTouchScroll: integer
- Enable or disable content touch-swipe scrolling for touch-enabled devices.
To completely disable, setcontentTouchScroll: false
. Integer values define the axis-specific minimum amount required for scrolling momentum (default value is25
).
markup:{ thumbnailsContainer: "string" }
- Set the element containing your thumbnails. By default, this is an unordered list (
ul
).
markup:{ thumbnailContainer: "string" }
- Set the element containing each thumbnail. By default this is a list-item (
li
).
markup:{ thumbnailElement: "string" }
- Set the actual thumbnail element. By default, this is an image tag (
img
).
advanced:{ autoExpandHorizontalScroll: boolean }
- Auto-expand content horizontally (for
"x"
or"yx"
axis).
If set totrue
(default), content will expand horizontally to accomodate any floated/inline-block elements (such asli
,a
,img
etc.).
advanced:{ updateOnContentResize: boolean }
- Update scroller(s) automatically on content, element or viewport resize. The value should be
true
(default) for fluid layouts/elements, adding/removing content dynamically, hiding/showing elements etc.
advanced:{ updateOnImageLoad: boolean }
- Update scroller(s) automatically each time an image inside the element is fully loaded. The value is
true
by default.
advanced:{ updateOnSelectorChange: "string" }
- Update scroller(s) automatically when the amount and size of specific selectors changes.
Useful when you need to update the scroller(s) automatically, each time a type of element is added, removed or changes its size.
For example, settingupdateOnSelectorChange: "ul li"
will update the scroller each time list-items inside the element are changed. Setting the value totrue
, will update the scroller each time any element is changed. To disable (default) set tofalse
.
theme: "string"
- Set a ready-to-use scroller theme (plugin’s CSS contains all themes).
callbacks:{ onInit: function(){} }
- A function/custom code to execute when the scroller has initialized (callbacks demo).
Example:
callbacks:{ onInit:function(){ console.log("scroller initialized"); } }
callbacks:{ onScrollStart: function(){} }
- A function/custom code to execute when scrolling starts (callbacks demo).
Example:
callbacks:{ onScrollStart:function(){ console.log("scroll started"); } }
callbacks:{ onScroll: function(){} }
- A function/custom code to execute when scrolling is completed (callbacks demo).
Example:
callbacks:{ onScroll:function(){ console.log("scroll completed"); } }
callbacks:{ onTotalScroll: function(){} }
- A function/custom code to execute when scrolling is completed and content is scrolled all the way to the end (bottom and/or right) (callbacks demo).
Example:
callbacks:{ onTotalScroll:function(){ console.log("Scrolled to 100%"); } }
callbacks:{ onTotalScrollBack: function(){} }
- A function/custom code to execute when scrolling is completed and content is scrolled back to the beginning (top and/or left) (callbacks demo).
Example:
callbacks:{ onTotalScrollBack:function(){ console.log("Scrolled back to 0%"); } }
callbacks:{ onTotalScrollOffset: integer }
- Set an offset for the onTotalScroll callback option.
For example, settingonTotalScrollOffset: 100
will trigger the onTotalScroll callback 100 pixels before the end of scrolling is reached.
callbacks:{ onTotalScrollBackOffset: integer }
- Set an offset for the onTotalScrollBack callback option.
For example, settingonTotalScrollBackOffset: 100
will trigger the onTotalScrollBack callback 100 pixels before the beginning of scrolling is reached.
callbacks:{ whileScrolling: function(){} }
- A function/custom code to execute while scrolling is active (callbacks demo).
Example:
callbacks:{ whileScrolling:function(){ console.log("scrolling..."); } }
callbacks:{ alwaysTriggerOffsets: boolean }
- Control the way onTotalScroll and onTotalScrollBack offsets execute.
By default, callback offsets will trigger repeatedly while content is scrolling within the offsets.
SetalwaysTriggerOffsets: false
when you need to trigger onTotalScroll and onTotalScrollBack callbacks only once.
live: boolean, "string"
- Enable or disable the creation of scroller(s) on all elements matching the current selector, now and in the future.
Setlive: true
when you need to add scroller(s) on elements that do not yet exist in the page. These could be elements added by other scripts or plugins after some action by the user.
If you need at any time to disable or enable the live option, setlive: "off"
and"on"
respectively.
You can also tell the script to disable live option after the first invocation by settinglive: "once"
.
liveSelector: "string"
- Set the matching set of elements to apply a scroller, now and in the future.
Plugin methods
Methods are ways to execute various scroller actions programmatically from within your script(s).
update
Usage $(selector).mThumbnailScroller("update");
Call the update method to manually update existing scrollers to accomodate new content or resized element(s). This method is by default called automatically by the script (via updateOnContentResize
option) when the element itself and/or its content size changes.
scrollTo
Usage $(selector).mThumbnailScroller("scrollTo", position, {options});
Call the scrollTo method to scroll content to the position parameter. Position parameter can be a string (e.g. "#element-id"
, "bottom"
, "left"
etc.), an integer indicating number of pixels (e.g. 100
), an array for y/x axis (e.g. [100,100]
), a js/jQuery object, a function etc. method demo
scrollTo position parameter
Position parameter can be:
"string"
- Element selector
For example, scroll to element with id “element-id”:
$(selector).mThumbnailScroller("scrollTo","#element-id");
- Special pre-defined position
For example, scroll to right:
$(selector).mThumbnailScroller("scrollTo","right");
Pre-defined position strings:
"bottom"
– scroll to bottom"top"
– scroll to top"right"
– scroll to right"left"
– scroll to left"first"
– scroll to the position of the first element within content"last"
– scroll to the position of the last element within content
- Number of pixels less/more: (e.g.
"-=100"
/"+=100"
)
For example, scroll by 100 pixels right:
$(selector).mThumbnailScroller("scrollTo","-=100");
- Element selector
integer
- Number of pixels (from left and/or top)
For example, scroll to 100 pixels:
$(selector).mThumbnailScroller("scrollTo",100);
- Number of pixels (from left and/or top)
[array]
- Different y/x position
For example, scroll to 100 pixels from top and 50 pixels from left:
$(selector).mThumbnailScroller("scrollTo",[100,50]);
- Different y/x position
object/function
- jQuery object
For example, scroll to element with id “element-id”:
$(selector).mThumbnailScroller("scrollTo",$("#element-id"));
- js object
For example, scroll to element with id “element-id”:
$(selector).mThumbnailScroller("scrollTo",document.getelementbyid("element-id"));
- function
For example, scroll to 100 pixels:
$(selector).mThumbnailScroller("scrollTo",function(){ return 100 });
- jQuery object
scrollTo method options
speed: integer
- Scrolling speed.
Example:
$(selector).mThumbnailScroller("scrollTo", "-=200", { speed: 30 });
duration: integer
- Scrolling animation duration, value in milliseconds.
Example:
$(selector).mThumbnailScroller("scrollTo", "right", { duration: 1000 });
easing: "string"
- Scrolling animation easing, values:
"easeInOut"
"easeOut"
"easeInOutSmooth"
"easeOutSmooth"
"easeInOutStrong"
"easeOutStrong"
"easeInOut"
"easeInOut"
Example:
$(selector).mThumbnailScroller("scrollTo", 300, { easing: "easeOutSmooth" });
callbacks: boolean
- Trigger user defined callbacks after scroll-to event is completed, values:
true
,false
.
Example:
$(selector).mThumbnailScroller("scrollTo", "top", { callbacks: false });
timeout: integer
- Method timeout (delay). The default timeout is 60 (milliseconds) in order to work with automatic scroller update functionality, value in milliseconds.
Example:
$(selector).mThumbnailScroller("scrollTo", "last", { timeout: 0 //no timeout });
stop
Usage $(selector).mThumbnailScroller("stop");
Stops running scrolling animations. Usefull when you wish to interrupt a previously scrollTo call.
disable
Usage $(selector).mThumbnailScroller("disable");
Calling disable method will temporarily disable the scroller(s). Disabled scrollers can be re-enabled by calling the update method.
To disable a scroller and reset its content position, call the method by setting its reset parameter to true
:
$(selector).mThumbnailScroller("disable", true);
destroy
Usage $(selector).mThumbnailScroller("destroy");
Calling destroy method will completely remove the scroller and return the element to its original state.
Scroller styling & themes
You can style your scroller(s) using jquery.mThumbnailScroller.css which contains the basic/default styling and few ready-to-use themes.
You can modify the default styling or an existing scroller theme directly, clone a theme and change it to your needs or overwrite the CSS in another stylesheet. If you wish to maintain compatibility with future plugin versions (that may include an updated version of jquery.mThumbnailScroller.css), I recommend using your own custom (or cloned) theme (creating your own custom theme), or overwriting the CSS. This way you can always upgrade jquery.mThumbnailScroller.css without having to redo your changes.
Themes
Plugin’s CSS includes a number of ready-to-use themes for quickly applying a basic styling to your scroller(s). To apply a theme, use theme
option parameter:
$(selector).mThumbnailScroller({ theme: "theme-name" });
or the HTML data attribute data-mts-theme
in your markup:
<div class="mThumbnailScroller" data-mts-theme="theme-name">...</div>
creating your own custom theme
User-defined callbacks
You can trigger your own js function(s) by using plugin’s callbacks option parameter (see all availbale callbacks in configuration section). Some examples:
$(selector).mThumbnailScroller({ callbacks:{ onScroll: function(){ /* do something */ } } });
$(selector).mThumbnailScroller({ callbacks:{ onTotalScroll: function(){ myFunction(); } } }); function myFunction(){ /* do something */ }
The plugin returns a number of values and objects that can be used inside the callbacks
this
– the scroller elementthis.mts.content
– the scrollable element containing the content (e.g. theul
element) as jquery objectthis.mts.top
– content’s top position (pixels)this.mts.left
– content’s left position (pixels)this.mts.topPct
– content vertical scrolling percentagethis.mts.leftPct
– content horizontal scrolling percentagethis.mts.direction
– content’s scrolling direction (returns"x"
or"y"
)
Plugin dependencies & requirements
- jQuery version 1.6.0 or higher
License
This work is released under the MIT License.
You are free to use, study, improve and modify it wherever and however you like.
https://opensource.org/licenses/MIT
Donating helps greatly in developing and updating free software and running this blog π
Thanks a lot for personally helping people who need help like us!
I have implemented the thumbnail scroller and Lightbox scripts which work great on Chrome, Safari and Firefox. However in Internet Explorer 8 the thumbnail scroller does not seem to function when I hover my mouse around. I tried getting down to the problem but without much luck. The only clue I have is that that the thumbnail scroller would work if I take out the Lightbox scripts.
Here is the webpage that I am currently working on: http://www.soiltrec.eu/newsdemo.html
I am new to javascript hence I would really appreciate some help in resolving this.
Many thanks
Tad
I’ll update the plugin and add some new features by Sunday. I’ll also modify some variable names along with the way ThumbnailScroller function is called. Hopefully, that will fix any conflict issues π
Done
Thanks a lot Malihu, I like the improvements and additional changes you have made especially the clickButton feature which was in my wishlist.
I have implemented the new thumbnail scroller (in addition to the Lightbox scripts) into the webpage below:
http://www.soiltrec.eu/newsdemo2.html
However the issue of the thumbnails not moving still seems to prevail in Internet Explorer. The jQuery.noConflict() function does solve the
script conflict issue in Chrome, Firefox and Safari, but the problem seems to be IE-specific which baffles me. Sometimes I wish IE never exist :/
@Tad
here: http://manos.malihu.gr/tuts/jts/with_lightbox/ you can see the demo of the plugin integrated with lightbox. The lightbox I used is version 2 (http://www.huddletogether.com/projects/lightbox2/) and it works well in all browsers including ie without any conflicts.
Thanks for getting back to me with an actual working webpage, it definitely helps. I suspect I might have accidentally made some changes which caused the thumbnail scroller not to work in ie. Now what I need to do is looking through my code (and comparing it side by side with yours) to see what went wrong.
Thanks again for the update today, the thumbnail scroller has just gotten better.
I found out that I was using a old/modified jquery script, changing that solves the problem.
@Tad
cool π
Love it. I’m in a basic html 5 class building a site.
My goal is to make the site phone friendly.
At one point in your comments you mentioned that you would look into creating the option to add up and down arrows to help navigate the scroller in ios environments.
Where you able to implement arrows?
Thanks
Jonathan
I have an arrows scroller ready somewhere although I’ve never posted it on the blog (don’t ask :P). I’ll check if I can implement it as an additional feature in this plugin by Sunday when I update it.
I’ve implemented next/previous buttons on the latest update of the plugin.
Without downloading and delving into this, can this lovely scroller be set to automatic scrolling? I don’t see that in the presentational code here. Thanks.
There’s no auto-scrolling feature at the moment. If you wanna delve into this, you can try to animate .container div ($thumbScroller_container.stop().animate()) based on its dimensions when mouse is off the thumbscroller.
Hi malihu,
Awesome scroller.
I have a similar request for auto scroll. I don’t know JS well enough to do this solo, would you be interested in adding that?
If so how much would it cost?
@vlad
I’ll try to get some time to update the plugin within this weekend and add auto-scroll feature, so it’d be great if you can wait till Sunday.
There’s no cost for updating or adding features to my scripts/plugins (I only charge fees for specific customization requests by users or clients). You can of course make a donation (“buy me a coffee” link) if you wanna support the development and updating of scripts or just feel like I helped in some way π
Added auto-scrolling feature to the plugin
Awesome major thanks, after this project is finished and I get paid I am sending you coffee $ for sure. Where are you located, that will depend on how much I send, cause coffee in NYC is probably like $20 and coffee in Bangkok is about $1 LOL j/k
Please check on our old “friend” IE 7, there is a weird glitch where everything jumps with the cursor. π
Thanks!
V
@Vlad
I’m located in Greece (Europe). Coffee is like 5-10 euro here but you can donate any amount you want really π Thanks.
Me, like everyone else, love what the script does and thank you Malihu for personally providing support to those who needed it.
I have a bit of a hiccup while implementing the script and I hope you can help me out with it.
Here is the webpage that I am currently working on: http://www.soiltrec.eu/newsdemo.html. I have also included Lightbox into the script which works great with your script in Safari, Chrome and Firefox. However it does not seem to run in IE8.
I tried getting down to the problem but without much luck. The only thing I found is that if I were to remove the Lightbox scripts, the thumbnail scroller script would work, which seem to suggest a conflict between scripts. However I have already put in the jQuery.noConflict() function so it seems to be a dead end there π Help is greatly apppreciated!
I’ve updated the code so check the latest plugin version
and how to do so when the mouse in img. img zoom
please help.
not running your script. what am I doing wrong?
http://www.grand-prix.ru/catalog/sistemi-videonabludenia.php
Seems to work now
This is SO AWESOME, thank you!!
Question: I’d like to do an image gallery like your “Denebola” and “Mark Sebastian” examples, with the old “click on the thumbnail to see the full size photo” routine. However, my site is more suited for a Vertical thumbnail bar than a horizontal bar, as you can see: http://www.whitefort.net/pix.html
I have no clue how to code it so it can figure out which thumbnail is being clicked upon–all the examples using OnClick-ey situations have horizontal scrollbars.
Li’l help?? Do you know of any examples or tutorials for vertical scrolling action? I don’t know much about javascript except it looks a lot like C+, which I learned, oh, 20-odd years ago and never used (being partial to Fortran, meself…)
Hey,
Thanks for this plugin. You have done it really well! Unfortunately we have experienced problems in internet explorer…
Please have a look at this website:
http://www.milieudesign.co.uk/index.php?webpage=product_detail.php&product_id=49359&cID=15981
In chrome and mozilla looks great but in internet explorer 8 it goes really funny.
Do you know what is wrong? I tried to add “style=”width: 960px!important;”” to the and it made it smaller but for some reason it stretches the content… It is just not working correctly…
I added small script to yours which magnifies thumbnails on hover. It doesnt cause the problem because I tried without this and it still have problems… But if you want I could provide you code to do that magnification so that you could implement it in you script.
Thanks for your help,
Chris
In ie 7 when you place your cursor over the thumbnails they disappear.
How can I fix this?
Is there a limit on the amount of thumb images you can load into this scroller? I have around 150 small thumbs (50×50), I can see the very last one fine but when I scroll all the way to the beginning the very first one seems to be hiding to the left to far.
Any ideas why?
Great tool btw!!!!
Respectfully,
Len
Hi,
I had this problem too, I tried to change things in “jquerry.thumbnailScroller.js” and it seems to work when you put 3 instead of 2 in this line (line 64 for me) of the function MouseMove :
var destY=-(((($outer_container.data(“totalContent”)+(tsMargin*2))-tsHeight)-tsHeight)*(mousePercentY));
I hope it helps you…
By the way, I’m looking for the same layout than Nick :
“I have a vertical scroller on the left and want images to display on the right. Any ideas?”
Very good job Malihu, I’ll have a look at your work more often.
Thank you !
Ok I was wrong, you just have to change figures in the $(window).load(function().
Thanks a lot for the wonderful script!
I tried to add lightbox animation for the images through connecting jquery.lightbox script in the and writing in the code ββ. But it doesnβt work. Is it something wrong in my actions or it is scripts incompatibility? Can you advise me what to do?
Check the latest version of the plugin
Hi,
I am new to jquery but have loaded a few on to my site and understand the process. I’m having trouble with all of my thumbnails showing with this plugin. Is there a max or min setting for number of thumbnails shown on a single line? I have 7 images total (but plan on adding several others) and only four show up on one line. I expanded the height and can see that the other three are bumped down to a separate row. This made me think this was a sizing issue, so I tried expanding the width of the container. Even though it was significantly wider, still only four thumbnails.
I also then tried shrinking down the thumbnails themselves. After they were pretty small (100px by 100px) six were shown on the top line but one was still below it.
I’m not sure if there is a setting to change this, but everything else is working perfectly and I really need to figure this out after the time I’ve spent getting to this point π
Thank you for your help!
Can you send me link so I can check it?Ooops just saw your e-mail π
Is there anyway to determine the opacity over the pictures, or how faded it is to begin with until you scroll over? It’s just a little too light for us, and we’d like to lessen the affect just a little bit.
Thanks!
@eRINN
The 6th parameter of the ThumbnailScroller function sets the default opacity of the thumbnails (values 0-1).
good work… but i got in ie6 error
Not supporting ie6 at all, sorry.
I am currently using this thumbnail scroller for my gallery on my website. Once I got the scroller working, it has performed beautifully. But I have one question.
Is there a way to integrate a function where when an image in the scroller is clicked, it is displayed is a pre-defined image area. I have found this code:
$(“#myImage”).attr(“src”, “path/to/newImage.jpg”);
but I am not sure that is what I want. I am somewhat of a jquery/java rookie. I have a vertical scroller on the left and want images to display on the right. Any ideas?
I’v had a problem with $thumbScroller_container width under IE and FireFox. Last .content div didn’t fit inside .container div. My solution for that was simply – I added +1 at this line:
$outer_container.data(“totalContent”,$outer_container.data(“totalContent”)+$(this).width()+1);
For me it made success. http://www.bener.com.pl/
Love the scroller but have a consistent problem with every scroller I have tried to use. When creating the scroller everything works great, when I use .load to load that page into a div on the main page the scroller breaks. I have tried loading thumbnail scroller with the .load callback function but no joy. This is not limited to your scroller it seems to be the same with *any* scroller I try. I would prefer to use yours! The other plugins on the same .load page work fine. Any ideas?
Need to see a link to check what exactly you wanna do
please check http://www.triasindo-jaya.com/malihu/
and i’ve upload the file http://www.triasindo-jaya.com/malihu/scrollertest.zip
thx
wrong post. sorry π
I’m having a weird issue in IE – http://ohmibod.com/index-update.html
The thumbnail scroller is blowing up:( It works great in FF & Chrome.
I’m using PrettyPhoto.js for a lightbox & jquery.cycle.min to run a slideshow.
Been staring at this all morning, any help would be appreciated!
Hi i use your scroller plugin and it works perfectly.
1. U said in you comment reply before that ur plugins can be combined with lightbox. I use this lightbox: http://www.huddletogether.com/projects/lightbox2/
and combine with your plugins but i can’t see it can work together.
this is my file http://www.triasindo-jaya.com/scrollertest.zip
2. What jquery captify plugin that can be combine to ur plugin?
As information, im trying to combine thumbnail scroller+captify+lightbox.
I really need your suggestion n help
Thanks a lot.
Hello Daffy,
In the address http://manos.malihu.gr/tuts/jts/with_lightbox/ I have implemented the plugin with the lightbox version 2 (the same lightboxyou use). It works as it should without any problems. You just need to download and implement the updated version of thumbnail scroller π
Thank u so much. You are my hero. I will download it as soon as I wake up tomorrow. U make me so happy today..
hi Malihu, I don’t find the download link/button on link u gave me (http://manos.malihu.gr/tuts/jts/with_lightbox/ ) so i try to “save page as” and change the path for js and everything needed to be change and then i dont see lightbox works. please suggest..
Thanks
Dear Malihu,
can u send me your http://manos.malihu.gr/tuts/jts/with_lightbox/ source code to my email?
i already download ur latest thumbnail scroller plugin and combine it to lightbox either i “save page as” that webpage and make change necessary but it doesn’t seem to work.
I don’t know my mistakes..
please help and thank u so much in advance…
Daffy
Daffy, I’ve uploaded a zip with all scroller and lightbox files. Download it here:
http://manos.malihu.gr/tuts/jts/with_lightbox/jts_with_lightbox.zip
Thank you so much.. it works perfectly
Hi,
This script is briliant ! thanks for sharing.
I get a strange behavior on page load/reload :
the thumbnails are “following” mouse position (instead of going in the opposite direction).
Any idea of what can cause this ?
Hi,
First, thanks for that plug-in. It’s exactly what I needed and pretty nice.
Unfortunaly, I have an issue that make the plug-in usuable. Do you have an idea about why it only displays 4 thumbnails on 24 (my site), or 1 on 3, or 1 on 2, etc. (I made tests)? I was developping my website using Firefox displaying my development (from my hard disk) and it was very fine… But when I putted the site online, even on Firefox I see only 4 thumbnails on 24… On Safari, I only get 4 thumbnails, on a server or on the hard disk.
I made tests for hours to find the problem, but I have no clue. Maybe you have seen this before, so you can help.
Thanks,
Yann
Hi,
I’d have to check the code online somewhere to see if I can help. These kind of problems though are usually simple to solve, usually regarding mistyped urls, missing classes etc.
Yeah I am having the same problem. It seems like there is an issue with loading several images. When it loads the images, it may load a few of them, and then stops. Not sure why. Once I reload, it’s fine, however it does not work properly until all the images have been loaded in the browser.
I’ve been trying to figure this out all day.
Otherwise, great script. It’s really coming in handy.
Hey!
I am having the same issues. Have you figured out how to solve this problem?
Thanks in advance!
Great plugin really usefull!
But I was wondering if there was a possiblility of adding a scrollbar . To move the scroll with it. And keeping the move of the scroll with the cursor.
Thanks!
I don’t if it was clear, I hope so
Hello! I’ve made another plugin that uses scrollbars. You can check it at: http://manos.malihu.gr/jquery-custom-content-scroller. Let me know if this is what you’re looking for π
Actually, I have a gallery with some photo with the same height but a different width. And I want to keep the scroll with the movement of the cursor on the pictures. But I want to add a scrollbar like that other plugin.
Because if i understand your other plugin, the pictures have the same size. And I have picture with different size.
Both plugins support images with different dimensions π
I’m thinking of implementing this on a site I’m working on. One drawback is that I don’t always want it to start with the leftmost item. Is there a command that I can use as an onLoad event to make the scroller start X number of pixels to the right of its leftmost edge? That way I could effectively start it in the middle, with the possibility of scrolling off to either side.
Hello David,
There are a couple of ways to do what you want.
The first, is by setting the left or top values of .container div in jquery.thumbnailScroller.css. A negative value (e.g. left:-300px) would initially set the scroller to start with the thumbnail at 300 pixels.
Another way would be to automatically set your scrollers to initially appear in the middle of the scrolling area. For example on horizontal scrollers, you could do this by editing jquery.thumbnailScroller.js adding:
var initPos=($thumbScroller_container.width()-$outer_container.width())/2; $thumbScroller_container.css("left",-initPos);
above MouseMove function.
Hi Malihu!
I’ve tried what you suggested, and the end result is that I can no longer scroll to the right past the image I moved to be pinned to the left.
I do this:
posOne = 0 – $(“#id_of_the_image”).position()[‘left’];
$(“#scrollerBlock1 .jTscrollerContainer”).css(“left”, posOne);
And that image become the left-most item (perfect), but I can’t ever get to anything to the left of it.
Thoughts?
Never mind, I’m just rewriting the whole thing.
I got the arrows to do the right thing by measuring various positions and showing or hiding the prev and next buttons as needed, and instead of moving .jTscrollerContainer, I moved .jTscroller. worked like a charm.
I need to substantially change how this works in some other ways in order to support fixed size objects with borders that always need to fall in place, so I’m starting from scratch.
Great extension though, super-useful, and got me to do what I needed to do. π
This is an outstanding piece of jquery plugin. Awsome! Thanks for sharing.
I want to ask the same thing as Giovanna: Is there a way to slow down the speed of the scroll?
You told about: var animSpeed=600; I can’t find this variable in any of these files: jquery.thumbnailScroller.js or jquery.easing.1.3.js. So where is this variable? Because I want to slow down the left / right sliding.
Thank you
Thanks Mihai!
The “var animSpeed=600;” doesn’t apply any more since I’ve changed the original script to a plugin. The equivalent value is now the “The scroll easing amount” parameter in ThumbnailScroller function call.
This value changes the easing amount of the animation. The actual speed of the scroll is determined by the movement of the cursor over the thumbnails area.
Love it, Love it, Love it. Please i really need your help with something when i insert the div with the thumbnails into a div inside of my coda slider #where, onmousover the thumbnails disappear out of view toward the right-hand side.
please see test page here: http://element.co.za/testsites2011/mfbf/test-index.html
click on #where
it’s strange, it works at first then when i refresh the page it doesn’t… please any guidance would be greatly appreciated.
Hello,
Try placing <script type=”text/javascript” src=”js/jquery.thumbnailScroller.js”></script> after calling ThumbnailScroller functions. You’ve also you inserted $.fx.prototype.cur function twice.
Hi, wow THANKS for having a look , I’ve cleaned up a bit like you said. but i’m still getting similar error.
now after they slide out of view if you open Firebug to inspect and mouse over the DIV area where the thumbnails are they shoot back and work as they should. same thing happens in Chrome with…
any idea’s? (bare in mind i’m no script ninja just learning as i go)
M
I think there’s an issue with the codaSlider script that scroll the pages. Try to place the thumbnail scroller scripts (both) after your last script (that among others, calls the codaSlider). If this doesn’t work, you’d need to call ThumbnailScroller function each time WHEREbutton is clicked and the document scrolling is completed.
Thanks for all your help you’ve helped me fix it. it works. we rally appreciate it. take care.
You’re welcome, thanks π
Very nice ! Thank you so much Mahilu for this wonderful work.
I have noticed that it doesn’t work on iphones our ipads. Do you have some information to fix that ?
Regards,
Dominique
Hello! The script is based on the mousemove event, which I don’t really expect to work correctly (or at all) on iOS devices. The thing is, I rarely develop for specific devices such as iPads etc. as they’re pretty limited, so I don’t know any solutions, fixes etc.
In my mind, a simple solution would be to add a condition to check for iOS and apply something like a normal scrollbar (e.g. css overflow:auto) on the container.
Thank you Mlihu
Really neat plugin, Thanks Loads!
30/1/2011
Modified the plugin to scroll horizontally, images with different widths.
is the updated code what I get from the “download” button?
because I didn’t find it working with images of different widths even after this update.
Yes, the archive is updated so you should be getting the modified .js. I think that you probably getting the cached .zip.
Anyway, you can also grab the updated script from the demo:
http://manos.malihu.gr/tuts/jquery.thumbnailScroller.js
Hello! Fantastic scroller. Much appreciation for your hard work in putting it together.
Is there a way to control it with up and down arrows? Thanks ahead of time!
Kim : )
Hello Kim,
Thanks for your comments π
I’ll definitely create a scroller that works with arrows. I just haven’t decided if it’s gonna be a different plugin or an updated version of this one. I’ll post an update as soon as it’s ready.
Thanks! Very cool. Looking forward to it!
Hello does anyone have a clue on how to set a grid of thumbnails (with grow hover effect) and movement only on the column or row you selected?
any sugestion on other jquery scripts?
Thanks and congrats for this fantastic script π
Got it in the multiple scrollers demo π
Is it possible to add some infinite scroll effect?
Infinite scroll? Can you describe its functionality?
On a row with 5 thumbnails when I hover to the fifth thumbnail the next thumbnail would be the first one, it would be more like a cycle π
Not possible the way the plugin works. It would be a totally different script (more like slideshow than a scroller).
Hi all,
If someone could write how to change the width and height of the container to accomodate for smaller thumbs (eg 100X70 px.), I would be very grateful.
Thanks for a great gallery plugin.
Hello,
Everything you need to change is in jquery.thumbnailScroller.css.
For instance, if you want your thumbnails to have 15px top and bottom padding and 70px height, you should set:
#ts_container .thumbScroller, #ts_container .thumbScroller .container, #ts_container .thumbScroller .content{height:100px;}
your work is amazing so thank you. I work with shadowbox plugin alot and i was wondering if you knew how to implement it as traditional methods are not working. Thanks π
Hi Ryan,
Shadowbox requires just a rel attribute on the thumbnail if I recall correctly. There should not be any problems since lightbox plugins usually do not interfere with other scripts. Can you post a link so I can check it further?
Thanks very much got it sorted, i was missing out a tiny bit of code but now all sorted. Cheers π
I have the same trouble of Smayton.
Thx Malibu, the version of jquery.thumbnailScroller.js for Shaun works perfectly.
All the best…
Love the thing, but no matter what I do, if the content images have variable widths, it seems that something is taking the largest width and applying that to each .content item (which some are half the width of the largest. Therefore, when I mouse to the right of the screen (either with fixed width horizontal or full) I have a large blank area and the items scroll out of sight.
I have calculated and put sizes in the css and even each individual img, alternately to the .content class, alternately to the container.
Yes, you’re right. For the horizontal scroller to work, you need to have equal width images. The reason css width doesn’t work, is because the plugin calculates and sets the total width of the container changing the initial css value.
I’m trying to find some time to modify the script to accept images with various widths. In the meanwhile, you can download this:
http://manos.malihu.gr/tuts/jquery.thumbnailScroller.zip
which is a modified version of the plugin I did for another user (shaun) that pretty much does the trick.
Edit: Plugin updated to scroll images with different widths
Thanks! So far, so good.