jQuery thumbnail scroller
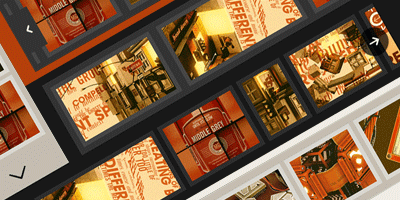
A thumbnail/image scroller that can be used as standalone or alongside lightboxes, gallery scripts etc. Features include: scrolling by cursor movement, buttons and/or touch, vertical and/or horizontal scrolling, customization via CSS and option parameters, methods for triggering events like scroll-to, update, destroy etc., user-defined callbacks functions and more.
Current version 2.0.3 (Changelog) – Version 1.x.x (no longer actively maintained)
How to use it
HTML
Get plugin files. Extract and upload jquery.mThumbnailScroller.min.js and jquery.mThumbnailScroller.css to your web server (more info)
Include jquery.mThumbnailScroller.css in the head tag your html document
<link rel="stylesheet" href="/path/to/jquery.mThumbnailScroller.css">
Include jQuery library (if your project doesn’t use it already) and jquery.mThumbnailScroller.min.js in your document’s head or body tag
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script> <script src="/path/to/jquery.mThumbnailScroller.min.js"></script>
Create the markup with your images, links, etc. which is basically an element (e.g. a div) holding an unordered list (ul)
<div id="my-thumbs-list"> <ul> <li><a href="image-1-link"><img src="/path/to/image-1-file" /></a></li> <li><a href="image-2-link"><img src="/path/to/image-2-file" /></a></li> <li><a href="image-3-link"><img src="/path/to/image-3-file" /></a></li> <li><a href="image-4-link"><img src="/path/to/image-4-file" /></a></li> <!-- and so on... --> </ul> </div>
CSS
Give your element (in the example above #my-thumbs-list) a width and/or height value and a CSS overflow value of auto or hidden. For example:
#my-thumbs-list{ overflow: auto; width: 800px; height: auto; }
If you’re creating a vertical scroller, you’ll need to set a height (or max-height) value
#my-thumbs-list{ overflow: auto; width: 300px; height: 500px; }
Initialization
Initialize via javascript
Create a script and call mThumbnailScroller function on your element selector along with the option parameters you want
<script> (function($){ $(window).load(function(){ $("#my-thumbs-list").mThumbnailScroller({ axis:"x" //change to "y" for vertical scroller }); }); })(jQuery); </script>
Initialize via HTML
If you prefer to initialize the plugin without a script, you can simply give your element the class mThumbnailScroller
and optionally set its orientation via the HTML data attribute data-mts-axis
("x"
for horizontal and "y"
for vertical). For example:
<div id="my-thumbs-list" class="mThumbnailScroller" data-mts-axis="x"> <ul> <!-- your content --> </ul> </div>
Basic configuration & option parameters
The 2 basic options are axis
which defines the scroller orientation and type
which defines how the user interacts with the scroller.
axis
The default scroller orientation is horizontal, so if you don’t set an axis
option value, it defaults to "x"
$("#my-thumbs-list").mThumbnailScroller({ axis:"x" });
To create a vertical scroller, set axis
option to "y"
$("#my-thumbs-list").mThumbnailScroller({ axis:"y" });
or use data-mts-axis="y"
if you’re initializing the plugin via HTML
type
You can set the type of scroller via javascript, for example:
$("#my-thumbs-list").mThumbnailScroller({ type:"hover-50" });
or using the HTML data attribute data-mts-type
, e.g.:
<div class="mThumbnailScroller" data-mts-type="hover-50">...</div>
There are 4 basic scroller types:
"hover-[number]"
(e.g."hover-33"
,"hover-80"
etc.)Scrolling content in linear mode by hovering the cursor over the scroller edges. The edges are defined by the type name, where [number] indicates the percentage of the scroller area in which scrolling is idle. For example, setting
"hover-50"
(default value) on a 600 pixels wide horizontal scroller, means that scrolling will be triggered only when the cursor is over the first 150 pixels from the left or right edge. And when cursor is over the 50% of the scroller width (300 pixels), scrolling will be idle or stopped.hover-precise
Scrolling content with animation easing (non-linear mode) by hovering the cursor over the scroller. The scrolling speed and direction is directly affected by the cursor movement and position within the scroller.
"click-[number]"
(e.g."click-50"
,"click-90"
etc.)Scrolling content by clicking buttons. The scrolling amount is defined by type name, where [number] indicates a percentage of the scroller area. For example, setting
"click-50"
on a 800 pixels wide horizontal scroller, means that each time you click the left or right arrow buttons, the content will scroll by 400 pixels (50% of 800px) to the left or right.click-thumb
Scrolling each image/thumbnail at a time by clicking buttons.
"hover-[number]"
and hover-precise
types become disabled on touch-enabled devices and replaced by content touch-swipe scrolling.
Configuration
You can configure your scroller(s) using the following option parameters when calling mThumbnailScroller function
Usage $(selector).mThumbnailScroller({ option: value });
setWidth: false
- Set the width of the scroller (overwrites CSS width), value in pixels (integer) or percentage (string).
setHeight: false
- Set the height of the scroller (overwrites CSS height), value in pixels (integer) or percentage (string).
setTop: 0
- Set the initial css top property of content, accepts string values (css top position).
Example:setTop: "-100px"
.
setLeft: 0
- Set the initial css left property of content, accepts string values (css left position).
Example:setLeft: "-100px"
.
type: "string"
- Scroller type defines the way users interact with the scroller.
Available values:"hover-[number]"
(e.g."hover-50"
)
Scrolling content in linear mode by hovering the cursor over the scroller edges. The edges are defined by the type name, where [number] indicates the percentage of the scroller area in which scrolling is idle. Scrolling accelerates proportionally according to cursor position: e.g. scrolling speed increases as the cursor moves towards the edges and decreases as it moves towards the center of the scroller area."hover-precise"
Scrolling content with animation easing (non-linear mode) by hovering the cursor over the scroller. The scrolling speed and direction is directly affected by the cursor movement and position within the scroller.click-[number]
(e.g."click-80"
)
Scrolling content by clicking buttons. The scrolling amount is defined by type name, where [number] indicates a percentage of the scroller area."click-thumb"
Scrolling each image/thumbnail at a time by clicking buttons. Each click will scroll the content by the width/height of the next non-visible adjusted image.
axis: "string"
- Define scroller axis (scrolling orientation).
Available values:"y"
,"x"
,"yx"
.axis: "y"
– vertical scrolleraxis: "x"
– horizontal scroller (default)axis: "yx"
– vertical and horizontal scroller (useful when panning images or switching axis on-the-fly)
speed: integer
- Set the scrolling speed (higher=faster). Default value is
15
.
contentTouchScroll: integer
- Enable or disable content touch-swipe scrolling for touch-enabled devices.
To completely disable, setcontentTouchScroll: false
. Integer values define the axis-specific minimum amount required for scrolling momentum (default value is25
).
markup:{ thumbnailsContainer: "string" }
- Set the element containing your thumbnails. By default, this is an unordered list (
ul
).
markup:{ thumbnailContainer: "string" }
- Set the element containing each thumbnail. By default this is a list-item (
li
).
markup:{ thumbnailElement: "string" }
- Set the actual thumbnail element. By default, this is an image tag (
img
).
advanced:{ autoExpandHorizontalScroll: boolean }
- Auto-expand content horizontally (for
"x"
or"yx"
axis).
If set totrue
(default), content will expand horizontally to accomodate any floated/inline-block elements (such asli
,a
,img
etc.).
advanced:{ updateOnContentResize: boolean }
- Update scroller(s) automatically on content, element or viewport resize. The value should be
true
(default) for fluid layouts/elements, adding/removing content dynamically, hiding/showing elements etc.
advanced:{ updateOnImageLoad: boolean }
- Update scroller(s) automatically each time an image inside the element is fully loaded. The value is
true
by default.
advanced:{ updateOnSelectorChange: "string" }
- Update scroller(s) automatically when the amount and size of specific selectors changes.
Useful when you need to update the scroller(s) automatically, each time a type of element is added, removed or changes its size.
For example, settingupdateOnSelectorChange: "ul li"
will update the scroller each time list-items inside the element are changed. Setting the value totrue
, will update the scroller each time any element is changed. To disable (default) set tofalse
.
theme: "string"
- Set a ready-to-use scroller theme (plugin’s CSS contains all themes).
callbacks:{ onInit: function(){} }
- A function/custom code to execute when the scroller has initialized (callbacks demo).
Example:
callbacks:{ onInit:function(){ console.log("scroller initialized"); } }
callbacks:{ onScrollStart: function(){} }
- A function/custom code to execute when scrolling starts (callbacks demo).
Example:
callbacks:{ onScrollStart:function(){ console.log("scroll started"); } }
callbacks:{ onScroll: function(){} }
- A function/custom code to execute when scrolling is completed (callbacks demo).
Example:
callbacks:{ onScroll:function(){ console.log("scroll completed"); } }
callbacks:{ onTotalScroll: function(){} }
- A function/custom code to execute when scrolling is completed and content is scrolled all the way to the end (bottom and/or right) (callbacks demo).
Example:
callbacks:{ onTotalScroll:function(){ console.log("Scrolled to 100%"); } }
callbacks:{ onTotalScrollBack: function(){} }
- A function/custom code to execute when scrolling is completed and content is scrolled back to the beginning (top and/or left) (callbacks demo).
Example:
callbacks:{ onTotalScrollBack:function(){ console.log("Scrolled back to 0%"); } }
callbacks:{ onTotalScrollOffset: integer }
- Set an offset for the onTotalScroll callback option.
For example, settingonTotalScrollOffset: 100
will trigger the onTotalScroll callback 100 pixels before the end of scrolling is reached.
callbacks:{ onTotalScrollBackOffset: integer }
- Set an offset for the onTotalScrollBack callback option.
For example, settingonTotalScrollBackOffset: 100
will trigger the onTotalScrollBack callback 100 pixels before the beginning of scrolling is reached.
callbacks:{ whileScrolling: function(){} }
- A function/custom code to execute while scrolling is active (callbacks demo).
Example:
callbacks:{ whileScrolling:function(){ console.log("scrolling..."); } }
callbacks:{ alwaysTriggerOffsets: boolean }
- Control the way onTotalScroll and onTotalScrollBack offsets execute.
By default, callback offsets will trigger repeatedly while content is scrolling within the offsets.
SetalwaysTriggerOffsets: false
when you need to trigger onTotalScroll and onTotalScrollBack callbacks only once.
live: boolean, "string"
- Enable or disable the creation of scroller(s) on all elements matching the current selector, now and in the future.
Setlive: true
when you need to add scroller(s) on elements that do not yet exist in the page. These could be elements added by other scripts or plugins after some action by the user.
If you need at any time to disable or enable the live option, setlive: "off"
and"on"
respectively.
You can also tell the script to disable live option after the first invocation by settinglive: "once"
.
liveSelector: "string"
- Set the matching set of elements to apply a scroller, now and in the future.
Plugin methods
Methods are ways to execute various scroller actions programmatically from within your script(s).
update
Usage $(selector).mThumbnailScroller("update");
Call the update method to manually update existing scrollers to accomodate new content or resized element(s). This method is by default called automatically by the script (via updateOnContentResize
option) when the element itself and/or its content size changes.
scrollTo
Usage $(selector).mThumbnailScroller("scrollTo", position, {options});
Call the scrollTo method to scroll content to the position parameter. Position parameter can be a string (e.g. "#element-id"
, "bottom"
, "left"
etc.), an integer indicating number of pixels (e.g. 100
), an array for y/x axis (e.g. [100,100]
), a js/jQuery object, a function etc. method demo
scrollTo position parameter
Position parameter can be:
"string"
- Element selector
For example, scroll to element with id “element-id”:
$(selector).mThumbnailScroller("scrollTo","#element-id");
- Special pre-defined position
For example, scroll to right:
$(selector).mThumbnailScroller("scrollTo","right");
Pre-defined position strings:
"bottom"
– scroll to bottom"top"
– scroll to top"right"
– scroll to right"left"
– scroll to left"first"
– scroll to the position of the first element within content"last"
– scroll to the position of the last element within content
- Number of pixels less/more: (e.g.
"-=100"
/"+=100"
)
For example, scroll by 100 pixels right:
$(selector).mThumbnailScroller("scrollTo","-=100");
- Element selector
integer
- Number of pixels (from left and/or top)
For example, scroll to 100 pixels:
$(selector).mThumbnailScroller("scrollTo",100);
- Number of pixels (from left and/or top)
[array]
- Different y/x position
For example, scroll to 100 pixels from top and 50 pixels from left:
$(selector).mThumbnailScroller("scrollTo",[100,50]);
- Different y/x position
object/function
- jQuery object
For example, scroll to element with id “element-id”:
$(selector).mThumbnailScroller("scrollTo",$("#element-id"));
- js object
For example, scroll to element with id “element-id”:
$(selector).mThumbnailScroller("scrollTo",document.getelementbyid("element-id"));
- function
For example, scroll to 100 pixels:
$(selector).mThumbnailScroller("scrollTo",function(){ return 100 });
- jQuery object
scrollTo method options
speed: integer
- Scrolling speed.
Example:
$(selector).mThumbnailScroller("scrollTo", "-=200", { speed: 30 });
duration: integer
- Scrolling animation duration, value in milliseconds.
Example:
$(selector).mThumbnailScroller("scrollTo", "right", { duration: 1000 });
easing: "string"
- Scrolling animation easing, values:
"easeInOut"
"easeOut"
"easeInOutSmooth"
"easeOutSmooth"
"easeInOutStrong"
"easeOutStrong"
"easeInOut"
"easeInOut"
Example:
$(selector).mThumbnailScroller("scrollTo", 300, { easing: "easeOutSmooth" });
callbacks: boolean
- Trigger user defined callbacks after scroll-to event is completed, values:
true
,false
.
Example:
$(selector).mThumbnailScroller("scrollTo", "top", { callbacks: false });
timeout: integer
- Method timeout (delay). The default timeout is 60 (milliseconds) in order to work with automatic scroller update functionality, value in milliseconds.
Example:
$(selector).mThumbnailScroller("scrollTo", "last", { timeout: 0 //no timeout });
stop
Usage $(selector).mThumbnailScroller("stop");
Stops running scrolling animations. Usefull when you wish to interrupt a previously scrollTo call.
disable
Usage $(selector).mThumbnailScroller("disable");
Calling disable method will temporarily disable the scroller(s). Disabled scrollers can be re-enabled by calling the update method.
To disable a scroller and reset its content position, call the method by setting its reset parameter to true
:
$(selector).mThumbnailScroller("disable", true);
destroy
Usage $(selector).mThumbnailScroller("destroy");
Calling destroy method will completely remove the scroller and return the element to its original state.
Scroller styling & themes
You can style your scroller(s) using jquery.mThumbnailScroller.css which contains the basic/default styling and few ready-to-use themes.
You can modify the default styling or an existing scroller theme directly, clone a theme and change it to your needs or overwrite the CSS in another stylesheet. If you wish to maintain compatibility with future plugin versions (that may include an updated version of jquery.mThumbnailScroller.css), I recommend using your own custom (or cloned) theme (creating your own custom theme), or overwriting the CSS. This way you can always upgrade jquery.mThumbnailScroller.css without having to redo your changes.
Themes
Plugin’s CSS includes a number of ready-to-use themes for quickly applying a basic styling to your scroller(s). To apply a theme, use theme
option parameter:
$(selector).mThumbnailScroller({ theme: "theme-name" });
or the HTML data attribute data-mts-theme
in your markup:
<div class="mThumbnailScroller" data-mts-theme="theme-name">...</div>
creating your own custom theme
User-defined callbacks
You can trigger your own js function(s) by using plugin’s callbacks option parameter (see all availbale callbacks in configuration section). Some examples:
$(selector).mThumbnailScroller({ callbacks:{ onScroll: function(){ /* do something */ } } });
$(selector).mThumbnailScroller({ callbacks:{ onTotalScroll: function(){ myFunction(); } } }); function myFunction(){ /* do something */ }
The plugin returns a number of values and objects that can be used inside the callbacks
this
– the scroller elementthis.mts.content
– the scrollable element containing the content (e.g. theul
element) as jquery objectthis.mts.top
– content’s top position (pixels)this.mts.left
– content’s left position (pixels)this.mts.topPct
– content vertical scrolling percentagethis.mts.leftPct
– content horizontal scrolling percentagethis.mts.direction
– content’s scrolling direction (returns"x"
or"y"
)
Plugin dependencies & requirements
- jQuery version 1.6.0 or higher
License
This work is released under the MIT License.
You are free to use, study, improve and modify it wherever and however you like.
https://opensource.org/licenses/MIT
Donating helps greatly in developing and updating free software and running this blog 🙂
Hi!
I was wondering where or which part can I change the design of the arrows?
also, how can I take the arrows outside so that it wouldn’t overlap the thumbnails.
Thanks!
1. You can use buttonsHTML option parameter to set the markup of each button, e.g.
markup:{ buttonsHTML:{ up:"your markup", down:"your markup", left:"your markup", right:"your markup" } }
or you could set an empty markup:
markup:{ buttonsHTML:{ up:"", down:"", left:"", right:"" } }
and add your arrows as background images in jquery.mThumbnailScroller.css (line 61: “2. SCROLLING BUTTONS STYLE”) or in your own stylesheet using the buttons selectors:
.mTSButtonUp .mTSButtonDown .mTSButtonLeft .mTSButtonRight
2. You can use the “buttons-out” theme to have them outside of the scroller area. Buttons CSS position is absolute so you can set their top, bottom, left and right values to anything you want.
You can see an example in demo.html in the second vertical scroller (#content-2):
https://github.com/malihu/thumbnail-scroller/blob/master/examples/demo.html#L239
Hi! Absolute love your plugin, I just have one question which really bothers my and I can’t seem to find it in the docs ;( (sorry, Dutchie)
How can I change the width of the area which triggers the move function? Or better yet: how can i remove the hover-to-move-scroller function (if I may call it like that) to an arrow whichs will move the scroller, not when u click it, but hover it 🙂
I want the same effects to be done, but just with arrows.
Hover arrow right: move scroll to right, etc etc
I can create the arrows myself, I just need to know how to destroy the hover function and build it again on the arrows.
Great job!
You can’t use the plugin arrows with hover. You can “disable” hover by setting type to “hover-100” (which means 100% of the element will render hover idle).
What you could do, is set it to something like “type: “hover-85” which means that scrolling will occur when the cursor is in the 15% (left/right) of the total width. Then you could insert your buttons under those 15% areas.
Hope I make sense lol
Haha, you did make sense!
That’s a lot better indeed, but I did actually make something to scroll the stuff to the right or left by using arrows.
Just a simple line:
$('.scrolLRight').hover(function(){ $('#scroller').mThumbnailScroller("scrollTo", "-=200"); });
That will move it like 200 pixels or whatever, but are you sure there is nothing to make with that to make it scroll continously just like the plugin does by itself?
The problem is that if I do use hover-85 the scroller wont scroll when I hover the arrow, because that is not on the same level as the scroller, its above it and wont trigger the scroller.
—
You can ignore above questions :), I changed the hover-85 to hover-97 and changed the z-index of the buttons which gives the illusion that you are scrolling by hovering the arrows.
Not the best solution but it works, thank you for your advice!
malihu,
Thank You for this excellent tool.
I need a little assistance. I am trying to setup in a SharePoint envoironment. In my initiall set-up, I had a fixed width and my fifth image wrapped around to the next ‘row’ below the first image.
I tweaked the width to 100% and it did not change anything. I have added and removed images and always the last image “wrap around” to the next row.
I looked through the CSS files (SharePoint and Scoller) and did not see antyhing.
Do you have any ideas or can suggest a setting to look at?
Thanks!
I removed
P.E.B.C.A.K.
First of all this is a lovely plugin and works very well…
However I have noticed that if using buttons to scroll the right button appears even if there is no content to scroll. It looks as though this shouldn’t be the case in the js file. How do I make it disappear if there is no content to scroll?
Thanks
Indeed. It seems that somehow I missed this…
To quickly fix this, edit jquery.mThumbnailScroller.css and change line 130 (https://github.com/malihu/thumbnail-scroller/blob/master/jquery.mThumbnailScroller.css#L130) from:
.mTSButton.mTS-hidden{
to:
.mTSButton.mTS-hidden, .mThumbnailScroller.mTS_no_scroll .mTSButton{
I’ll try to push this in a new plugin version asap.
Thanks a lot for the feedback 🙂
EDIT: CSS updated
Lovely, that’s fixed it!
hey sir
i implement this scroller it works fine,but i change it with my requirements, i use bootstrap panel on button click instead of image. when i click on button to add new panel in list, first panel should move left.
i used SCROLL-TO function that not work fine for me.
Any suggestion how can i do it.if you provide your email i will add image of my problem. Hope you will help me.
My contact info is in the contact me page. I’d need to see your page and/or code in order to help.
thank you sir.i send the email at you email address with sample code.
Hello, thanks for this plug in, really useful :).
I have 2 questions :
Is there a way to scroll using the wheel while the mouse is in the scroller ?
Is there a way to make the scroller elements circular ? (Put the first element after the last and the last before the first on scrolling)
Hi,
Carousel scrolling (circular elements) is not currently supported by the script (it can’t really work with the “hover-” scrolling types).
For the mousewheel, you could create a script and combine the jquery-mousewheel plugin with thumbnail scroller’s scrollTo method. For example, something like:
$("#my_elem").on("mousewheel", function(event) { if(event.deltaX>0){ $(selector).mThumbnailScroller("scrollTo","-=100"); }else if(event.deltaX<0){ $(selector).mThumbnailScroller("scrollTo","+=100"); } });
Hi.
is it possible that the scroller doesn’t works if there are less items to scroll?
I certainly wrong something…..
On a small monitor the scroller works correctly because the total width of all the items is greater than monitor width, but on widescreen monitor, if the total width of all the items is smallest (and the scroll doesn’t need), the scroller doesn’t starts. Appears only the preloader.
Thank you 🙂
This was a bug with version 2.0.1. I just fixed it and updated plugin to version 2.0.2, so just grab and use latest version:
https://github.com/malihu/thumbnail-scroller/releases
GGGGRRRREEEAAAT Malihu!!!!
It works perfectly.
Thank you 🙂
Great plugin.
Is there any way to open images in full screen like lightbox gallery with previus and next button when user click on the image ?
This is out of plugin’s scope but you can use any gallery/lightbox plugin along with this script easily.
When I call $(“#slider-element”).mThumbnailScroller(“destroy”);
Elements that were not created by the plugin are removed. I append new elements to the slider after the plugin is initiated. When I call “destroy” I would expect it to only remove elements, classes, attr, and other content created by the plugin. It instead removes that and all other elements that I appended within the slider.
How can I only have the “destroy” only remove elements created by the plugin?
Any solution?
You are correct. This is a script limitation at the moment. I’ll try fixing this on the next version (2.0.3). In the meantime, maybe you could use the disable method instead?
I need the elements created by the plugin to be removed from the DOM. When do you expect to release the next update? I can wait.
Hi, that’s a great job.
I just would like to set up the auto play (linear) and pause when the mouse is on.
I’ve tried what’s proposed on http://manos.malihu.gr/repository/jquery-thumbnail-scroller/demo/examples/auto_scroll_example.html
Mine is vertical so I’ve changed left and right by to and bottom but it doesn’t work either.
May you have an idea.
Thanks a lot
Karen
Hello,
Have you set axis option to “y”? Have you changed the CSS width and height values for your vertical scroller accordingly?
The following code is working on vertical scrollers:
var loopDuration=6000; $("#content-1").mThumbnailScroller({ axis:"y", callbacks:{ onInit:function(){ $("#content-1").mThumbnailScroller("scrollTo","bottom",{duration:loopDuration/2,easing:"linear"}); }, onTotalScroll:function(){ if($(this).data("mTS").trigger==="external"){ $("#content-1").mThumbnailScroller("scrollTo","top",{duration:loopDuration/2,easing:"linear"}); } } } });
I would like to use your scroller in the following way:
–vertical scrolling of images
–autoplay like on your page: http://manos.malihu.gr/repository/jquery-thumbnail-scroller/demo/examples/auto_scroll_example.html
–autoplay continuously (never stop), unless hovered on an image.
Can this be done?
With Joomla 3 causes compatibility problems with other libraries.
Maybe you need to use:
jQuery.noConflict();
in your script(?)
See more info here:
http://learn.jquery.com/using-jquery-core/avoid-conflicts-other-libraries/
Is not it easier to use it you?
Hey Malihu
thank you for your last suggestion,i am again in trouble,i want to use this jquery thumbnail scroller in Angularjs.i tried to use it,but it working fine in google chrome..but not working on responsive view and also not on mozila firefox.
But if i use it in simple project it works perfectly…
is there any way to use it in angularjs..
hope you got the point..
Regards
Ahsan Mustafa
Hi Malihu.
Great work!
My question is:
is it possible to start scrolling the list by center elements and not by the first? How?
Thank you.
Hello,
Without knowing what you’re trying to do, have you checked the type option parameter?
Maybe you need something like:
$(selector).mThumbnailScroller({ type: "hover-20" });
Hi.
Those parameters are used to manage the movement (sensible area and event) of thumbnails, correct?
But, simply, I would like to center the strip of thumbnails and start to move from the center of the slide thumbnails, not from the first element.
Thank you.
Then you should use setLeft option paraneter (or setTop for vertical scrollers) to set the initial CSS left property of the content.
Is this what you need?
YES! 🙂
Thank you Malihu!
I want to add carousel controls to small thumbnail in bootstrap carousel
I don’t really understand what you’re trying to do… Do you want to combine bootstrap carousel with thumbnail scroller plugin? If yes, in what manner? Both are sliders/scrollers having similar functionality.
<div id="myCarousel" class="carousel slide article-slide"> <div class="carousel-inner cont-slider"> <div class="item active"><img class="alignnone size-full wp-image-335" src="http://www.studio.ayson.com/wp-content/uploads/2015/01/116.jpg" alt="1" width="1024" height="400" /></div> <div class="item"><img class="alignnone size-full wp-image-336" src="http://www.studio.ayson.com/wp-content/uploads/2015/01/25.jpg" alt="2" width="1024" height="400" /></div> <div class="item"><img class="alignnone size-full wp-image-337" src="http://www.studio.ayson.com/wp-content/uploads/2015/01/35.jpg" alt="3" width="1024" height="400" /></div> <div class="item"><img class="alignnone size-full wp-image-338" src="http://www.studio.ayson.com/wp-content/uploads/2015/01/45.jpg" alt="4" width="1024" height="400" /></div> <div class="item"><img class="alignnone size-full wp-image-339" src="http://www.studio.ayson.com/wp-content/uploads/2015/01/55.jpg" alt="5" width="1024" height="400" /></div> <div class="item"><img class="alignnone size-full wp-image-340" src="http://www.studio.ayson.com/wp-content/uploads/2015/01/65.jpg" alt="6" width="1024" height="400" /></div> <div class="item"><img class="alignnone size-full wp-image-341" src="http://www.studio.ayson.com/wp-content/uploads/2015/01/75.jpg" alt="7" width="1024" height="400" /></div> </div> <div class="slick"> <ol class="carousel-indicators visible-lg visible-md"> <li class="active" data-slide-to="0" data-target="#myCarousel"><img class="alignnone size-full wp-image-250" src="http://www.studio.ayson.com/wp-content/uploads/2015/01/1.jpg" alt="1" width="60" height="60" /></li> <li class="" data-slide-to="1" data-target="#myCarousel"><img class="alignnone size-full wp-image-251" src="http://www.studio.ayson.com/wp-content/uploads/2015/01/2.jpg" alt="2" width="60" height="60" /></li> <li class="" data-slide-to="2" data-target="#myCarousel"><img class="alignnone size-full wp-image-252" src="http://www.studio.ayson.com/wp-content/uploads/2015/01/3.jpg" alt="3" width="60" height="60" /></li> <li class="" data-slide-to="3" data-target="#myCarousel"><img class="alignnone size-full wp-image-253" src="http://www.studio.ayson.com/wp-content/uploads/2015/01/4.jpg" alt="4" width="60" height="60" /></li> <li class="" data-slide-to="4" data-target="#myCarousel"><img class="alignnone size-full wp-image-254" src="http://www.studio.ayson.com/wp-content/uploads/2015/01/5.jpg" alt="5" width="60" height="60" /></li> <li class="" data-slide-to="5" data-target="#myCarousel"><img class="alignnone size-full wp-image-255" src="http://www.studio.ayson.com/wp-content/uploads/2015/01/6.jpg" alt="6" width="60" height="60" /></li> <li class="" data-slide-to="6" data-target="#myCarousel"><img class="alignnone size-full wp-image-256" src="http://www.studio.ayson.com/wp-content/uploads/2015/01/7.jpg" alt="7" width="60" height="60" /></li> </ol> </div> <div class="bs_controls"><a class="left carousel-control" href="#myCarousel" role="button" data-slide="prev"><img src="http://www.studio.ayson.com/wp-content/uploads/2015/01/left_arr1.png" alt="left_arr" width="18" height="27" class="alignnone size-full wp-image-383" /><span class="sr-only">Previous</span></a> <a class="right carousel-control" href="#myCarousel" role="button" data-slide="next"><img src="http://www.studio.ayson.com/wp-content/uploads/2015/01/rightarr1.png" alt="rightarr" width="20" height="19" class="alignnone size-full wp-image-384" /><span class="sr-only">Next</span></a><div class="playpause"> <a class="right" id="playButton" > <img src="http://www.studio.ayson.com/wp-content/uploads/2015/01/bdarrow.png" alt="bdarrow" width="7" height="9" class="alignnone size-full wp-image-419"/> </a> <a class="right1" id="playButton" > <img src="http://www.studio.ayson.com/wp-content/uploads/2015/01/bdarrow1.png" alt="bdarrow" width="7" height="9" class="alignnone size-full wp-image-419"/> </a><a class="left" id="pauseButton"> <img src="http://www.studio.ayson.com/wp-content/uploads/2015/01/pau_arr.png" alt="pau_arr" width="17" height="24" class="alignnone size-full wp-image-389" /> </a> </div> </div><!---slick---> </div> </div> </div>
sorry this is jquery
(function($){
$(window).load(function(){
$(“#my-thumbs-list”).mThumbnailScroller({
axis:”x”, //change to “y” for vertical scroller
});
});
})(jQuery);
Bootstrap code not displaying here
Hi, i’m trying to use your plugin for my website, which works great, so i decided starting tests on mobile devices, specifically android tablets (i’ll try ipads onwards) and i have a little issue: I have a main div 500% wide, with 5 sections each one 20% wide, every desktop browser fits each 20% section to screen width, which looks and works great . However, when i open the file on any android browser, it fits and shows the entire 500% div width on screen, obviously the entire layout looks large and thin. At this point i’m still working with local files. What am i doing wrong? Is it a compatibility issue? Thank you so much.
I can’t really say but as far the plugin goes, you could try disabling autoExpandHorizontalScroll option parameter (set to false or 0) and see if works for you.
I couldn`t find a way to make it work as i needed to, so i ended up using another plugin, but thank you so much anyway.
Sir is there possible to move image like normal slider
not move whole image list..
Yes.
Setting the type option parameter to “click-thumb” scrolls each image/thumbnail at a time (like the top scroller in this demo).
Another way would be to give your images the same width as your container element and set type to “click-100”.
Another great plugin, Is this slider limited to just images or can I put anything in it for sliding?
You can slide anything.
You can define your own element selectors (see markup options in “Configuration”). More specifically, you should define what your actual “thumbnail” element is using the thumbnailElement option parameter. For example, if you would like to slide paragraphs inside unordered list items you should do:
$("#content-1").mThumbnailScroller({ markup:{ thumbnailElement: "p" } });
Using the markup options you can really slide anything 😉
removing the that contain each thumbnail leaves empty space when removing that it still scrolls to when theres nothing there. Does this work well with appending and removing content?
Yes. Can you send me link/code to understand what you’re trying to do?
Hey Malihu,
you’ve created great pieces of code.
How can we turn the thumbnail scroller into a kind of endless scroller?
Thank you in advance
You can’t (at least at the moment) and carousel-like scrolling would only work with “click” scroller types. I’ll try to add such feature on the next version.
Hey Malihu
thank you for your last suggestion,i am again in trouble,i want to use this jquery thumbnail scroller in Angularjs.i tried to use it,but it working fine in google chrome..but not working on responsive view and also on mozila firefox.
is there any way to use it in angularjs..
hope you got the point..
Regards
Ahsan Mustafa
Hi and thanks for the great extension.
Would you be able to tell me how to reverse the direction of the scrolling? I have a RTL site and I have put RTL in my css but the scroller doesnt scroll the right way so it goes too far to the right and not enough to the left?
Thanks in advance.
Hello,
In order for the script to work correctly without any other CSS changes, apply the RTL direction using the following ways:
1. via HTML
Add dir=”rtl” to your root element, for example:
<body dir="rtl">
2. via CSS
Add “direction: rtl” to any parent element, e.g.:
body{ direction: rtl; }
In any case, do not apply the direction on the scroller element directly. Just apply it to a parent element.
Hi,
first of all, thanx for the great job of this scroller.
my issue is: i’ve like to use this scroller with the yx axis, depend on media queries.
your demo of this works very well. but my layout needs a scroll x on smaller widths(horizontal bar 100%), and a scroll y on larger widths (vertical sidebar). i tried some changes in the css, but i can’t figure it out.
is there a simple solution for?
grtz,
Thom.
“Reversing” the CSS properties in css_media_queries_demo.html for example will do exactly what you need. Change the CSS of the demo to the following and test it:
.content{ overflow: auto; position: relative; padding: 10px; background: #333; margin: 10px; width: 65%; height: auto; float: none; } .content li{ float: left; margin: 0 4px; overflow: hidden; } .content li a{ display: inline-block; border: 7px solid rgba(255,255,255,.1); } .content .mTSButton{ background-color: #333; } @media only screen and (min-width: 1024px){ .content{ width: 325px; height: 500px; float: left; } .content li{ float: none; margin: 8px 0; } }
Ok, that did the trick
Thnx for the quick support !
Thom.
Dude great tutorial and plugin really help me a lot.
One quick question, i don’t get how to make the scroller load or start when it is ready.
Right now it take a sec for the scroller to get ready. You can see a jump in the page when loading.
Thank you
Have you checked show_when_ready_example.html in plugin archive? You can see it online here: http://manos.malihu.gr/repository/jquery-thumbnail-scroller/demo/examples/show_when_ready_example.html
Normally, if your element has a height value set and it’s
overflow: hidden;
you won’t see the “jump”.Hi, great script!
It’s possible to “autoplay the scroll when the page is loaded and pause it when we are hover?
Thanks a lot!
Sure. Take a look at the auto_scroll_example.html in plugin archive. It does exactly what you describe with few lines of code (see it online: http://manos.malihu.gr/repository/jquery-thumbnail-scroller/demo/examples/auto_scroll_example.html).
Thank for the answar!
I’ve a just last question..
I’ve implemented this solution but I need a sloowly and costant movement … and with your code the scroll accellerate in the middle.
I’ve tried to put “speed=1” but don’t work..
You just need to change the easing option in the scrollTo method call to
"linear"
. For example in auto_scroll_example.html:$("#content-1").mThumbnailScroller("scrollTo","right",{duration:loopDuration/2,easing:"linear"});
Thank you very much!
Hi there Malihu. Amazing work both this and the mcustom scrollbar!
I’m trying out this one now.. I setup everything and everything works just fine (using latest version of this plugin of course!). My only gripe is when using ‘hover-precise’. This mode should be integrated with the regular ‘hover-[number]’ functionality. By that, I mean, it should scroll all the way to the far end when I’m holding my mouse short of the scrolled container edge (currently, I have to drag my mouse after/before the container width and even then, end content won’t show up fully). Otherwise, content placed at the far ends of the container are half-showing. I noticed this ‘issue’ is persistent within your example so figured my configuration is correct.
I hope you can fix/add this functionality so UX improves to 100% when using ‘hover-precise’.
Thanks again!
Hello and thanks for the comments and feedback!
“hover-precise” type scrolls the content based on the exact position of cursor. For a better user experience when using this type, you normally need to add a left/right margin to your first and last elements. This will make scrolling on the edges much better.
I have a ready-to-use theme that does that automatically. Try using the “hover-full” theme (
theme: "hover-full"
) to see what I mean. You can see its CSS on github (see /* theme: “hover-full” */).Let me know if this helps
Indeed that worked better. However, I did like the smoothness of using ‘precise’. Is it possible to create a theme that acts like ‘full’ around the edges of the container and like ‘precise’ around the middle? I want to make scroll-ability more noticeable to the user.
“hover-full” theme uses the “hover-precise” type along with few other options. However, you could simply set the type to “hover-precise” and just add the margins to the first/last elements. For example:
#your-element-id .mTSThumbContainer:first-child{ margin-left: 40px; } #your-element-id .mTSThumbContainer:last-child{ margin-right: 40px; }
hey man! thanks for the thumbnail scroller plugin! loved it!
just wondering, there is some way to break the items in rows? I have a layout with this exigence and will be very nice have a option like “numRows”.
there is some way? and if there is not, think is a big improvement.
thanks again!
Hi,
There’s no way for the script to break list items into rows. You’ll need to create your layout that way, e.g. stacking divs or adding plugin markup inside existing list-items.
I can’t say if I’ll implement such feature as it’s a bit off plugin scope.
You might be able to accomplish what you need (without editing much or at all your existing HTML) by using plugin’s
markup
option parameter to define your own elements (see Configuration). I can’t say for sure if this would work as I’d need to see your HTML/layout.i used your slider in my magento store it will working perfectly. but there is one problem is there any way to make this slider responsive
See version 2.0.0 (just released)
Hi,
I have one page full of different product images. When you hover on an image you see thumbnail pictures of different colors of that products. I want to use your plugin for every image in page but only the first one works. How can i do that?
Hi. I implemented the scroller and it works great, but when I create a second scroller it only displays the first 4 thumbnails and doesn’t work. Only works one scroller
Any idea how can I have two identical scrollers? In the same page?
Thanks