jQuery thumbnail scroller
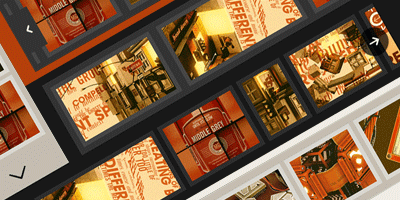
A thumbnail/image scroller that can be used as standalone or alongside lightboxes, gallery scripts etc. Features include: scrolling by cursor movement, buttons and/or touch, vertical and/or horizontal scrolling, customization via CSS and option parameters, methods for triggering events like scroll-to, update, destroy etc., user-defined callbacks functions and more.
Current version 2.0.3 (Changelog) – Version 1.x.x (no longer actively maintained)
How to use it
HTML
Get plugin files. Extract and upload jquery.mThumbnailScroller.min.js and jquery.mThumbnailScroller.css to your web server (more info)
Include jquery.mThumbnailScroller.css in the head tag your html document
<link rel="stylesheet" href="/path/to/jquery.mThumbnailScroller.css">
Include jQuery library (if your project doesn’t use it already) and jquery.mThumbnailScroller.min.js in your document’s head or body tag
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script> <script src="/path/to/jquery.mThumbnailScroller.min.js"></script>
Create the markup with your images, links, etc. which is basically an element (e.g. a div) holding an unordered list (ul)
<div id="my-thumbs-list"> <ul> <li><a href="image-1-link"><img src="/path/to/image-1-file" /></a></li> <li><a href="image-2-link"><img src="/path/to/image-2-file" /></a></li> <li><a href="image-3-link"><img src="/path/to/image-3-file" /></a></li> <li><a href="image-4-link"><img src="/path/to/image-4-file" /></a></li> <!-- and so on... --> </ul> </div>
CSS
Give your element (in the example above #my-thumbs-list) a width and/or height value and a CSS overflow value of auto or hidden. For example:
#my-thumbs-list{ overflow: auto; width: 800px; height: auto; }
If you’re creating a vertical scroller, you’ll need to set a height (or max-height) value
#my-thumbs-list{ overflow: auto; width: 300px; height: 500px; }
Initialization
Initialize via javascript
Create a script and call mThumbnailScroller function on your element selector along with the option parameters you want
<script> (function($){ $(window).load(function(){ $("#my-thumbs-list").mThumbnailScroller({ axis:"x" //change to "y" for vertical scroller }); }); })(jQuery); </script>
Initialize via HTML
If you prefer to initialize the plugin without a script, you can simply give your element the class mThumbnailScroller
and optionally set its orientation via the HTML data attribute data-mts-axis
("x"
for horizontal and "y"
for vertical). For example:
<div id="my-thumbs-list" class="mThumbnailScroller" data-mts-axis="x"> <ul> <!-- your content --> </ul> </div>
Basic configuration & option parameters
The 2 basic options are axis
which defines the scroller orientation and type
which defines how the user interacts with the scroller.
axis
The default scroller orientation is horizontal, so if you don’t set an axis
option value, it defaults to "x"
$("#my-thumbs-list").mThumbnailScroller({ axis:"x" });
To create a vertical scroller, set axis
option to "y"
$("#my-thumbs-list").mThumbnailScroller({ axis:"y" });
or use data-mts-axis="y"
if you’re initializing the plugin via HTML
type
You can set the type of scroller via javascript, for example:
$("#my-thumbs-list").mThumbnailScroller({ type:"hover-50" });
or using the HTML data attribute data-mts-type
, e.g.:
<div class="mThumbnailScroller" data-mts-type="hover-50">...</div>
There are 4 basic scroller types:
"hover-[number]"
(e.g."hover-33"
,"hover-80"
etc.)Scrolling content in linear mode by hovering the cursor over the scroller edges. The edges are defined by the type name, where [number] indicates the percentage of the scroller area in which scrolling is idle. For example, setting
"hover-50"
(default value) on a 600 pixels wide horizontal scroller, means that scrolling will be triggered only when the cursor is over the first 150 pixels from the left or right edge. And when cursor is over the 50% of the scroller width (300 pixels), scrolling will be idle or stopped.hover-precise
Scrolling content with animation easing (non-linear mode) by hovering the cursor over the scroller. The scrolling speed and direction is directly affected by the cursor movement and position within the scroller.
"click-[number]"
(e.g."click-50"
,"click-90"
etc.)Scrolling content by clicking buttons. The scrolling amount is defined by type name, where [number] indicates a percentage of the scroller area. For example, setting
"click-50"
on a 800 pixels wide horizontal scroller, means that each time you click the left or right arrow buttons, the content will scroll by 400 pixels (50% of 800px) to the left or right.click-thumb
Scrolling each image/thumbnail at a time by clicking buttons.
"hover-[number]"
and hover-precise
types become disabled on touch-enabled devices and replaced by content touch-swipe scrolling.
Configuration
You can configure your scroller(s) using the following option parameters when calling mThumbnailScroller function
Usage $(selector).mThumbnailScroller({ option: value });
setWidth: false
- Set the width of the scroller (overwrites CSS width), value in pixels (integer) or percentage (string).
setHeight: false
- Set the height of the scroller (overwrites CSS height), value in pixels (integer) or percentage (string).
setTop: 0
- Set the initial css top property of content, accepts string values (css top position).
Example:setTop: "-100px"
.
setLeft: 0
- Set the initial css left property of content, accepts string values (css left position).
Example:setLeft: "-100px"
.
type: "string"
- Scroller type defines the way users interact with the scroller.
Available values:"hover-[number]"
(e.g."hover-50"
)
Scrolling content in linear mode by hovering the cursor over the scroller edges. The edges are defined by the type name, where [number] indicates the percentage of the scroller area in which scrolling is idle. Scrolling accelerates proportionally according to cursor position: e.g. scrolling speed increases as the cursor moves towards the edges and decreases as it moves towards the center of the scroller area."hover-precise"
Scrolling content with animation easing (non-linear mode) by hovering the cursor over the scroller. The scrolling speed and direction is directly affected by the cursor movement and position within the scroller.click-[number]
(e.g."click-80"
)
Scrolling content by clicking buttons. The scrolling amount is defined by type name, where [number] indicates a percentage of the scroller area."click-thumb"
Scrolling each image/thumbnail at a time by clicking buttons. Each click will scroll the content by the width/height of the next non-visible adjusted image.
axis: "string"
- Define scroller axis (scrolling orientation).
Available values:"y"
,"x"
,"yx"
.axis: "y"
– vertical scrolleraxis: "x"
– horizontal scroller (default)axis: "yx"
– vertical and horizontal scroller (useful when panning images or switching axis on-the-fly)
speed: integer
- Set the scrolling speed (higher=faster). Default value is
15
.
contentTouchScroll: integer
- Enable or disable content touch-swipe scrolling for touch-enabled devices.
To completely disable, setcontentTouchScroll: false
. Integer values define the axis-specific minimum amount required for scrolling momentum (default value is25
).
markup:{ thumbnailsContainer: "string" }
- Set the element containing your thumbnails. By default, this is an unordered list (
ul
).
markup:{ thumbnailContainer: "string" }
- Set the element containing each thumbnail. By default this is a list-item (
li
).
markup:{ thumbnailElement: "string" }
- Set the actual thumbnail element. By default, this is an image tag (
img
).
advanced:{ autoExpandHorizontalScroll: boolean }
- Auto-expand content horizontally (for
"x"
or"yx"
axis).
If set totrue
(default), content will expand horizontally to accomodate any floated/inline-block elements (such asli
,a
,img
etc.).
advanced:{ updateOnContentResize: boolean }
- Update scroller(s) automatically on content, element or viewport resize. The value should be
true
(default) for fluid layouts/elements, adding/removing content dynamically, hiding/showing elements etc.
advanced:{ updateOnImageLoad: boolean }
- Update scroller(s) automatically each time an image inside the element is fully loaded. The value is
true
by default.
advanced:{ updateOnSelectorChange: "string" }
- Update scroller(s) automatically when the amount and size of specific selectors changes.
Useful when you need to update the scroller(s) automatically, each time a type of element is added, removed or changes its size.
For example, settingupdateOnSelectorChange: "ul li"
will update the scroller each time list-items inside the element are changed. Setting the value totrue
, will update the scroller each time any element is changed. To disable (default) set tofalse
.
theme: "string"
- Set a ready-to-use scroller theme (plugin’s CSS contains all themes).
callbacks:{ onInit: function(){} }
- A function/custom code to execute when the scroller has initialized (callbacks demo).
Example:
callbacks:{ onInit:function(){ console.log("scroller initialized"); } }
callbacks:{ onScrollStart: function(){} }
- A function/custom code to execute when scrolling starts (callbacks demo).
Example:
callbacks:{ onScrollStart:function(){ console.log("scroll started"); } }
callbacks:{ onScroll: function(){} }
- A function/custom code to execute when scrolling is completed (callbacks demo).
Example:
callbacks:{ onScroll:function(){ console.log("scroll completed"); } }
callbacks:{ onTotalScroll: function(){} }
- A function/custom code to execute when scrolling is completed and content is scrolled all the way to the end (bottom and/or right) (callbacks demo).
Example:
callbacks:{ onTotalScroll:function(){ console.log("Scrolled to 100%"); } }
callbacks:{ onTotalScrollBack: function(){} }
- A function/custom code to execute when scrolling is completed and content is scrolled back to the beginning (top and/or left) (callbacks demo).
Example:
callbacks:{ onTotalScrollBack:function(){ console.log("Scrolled back to 0%"); } }
callbacks:{ onTotalScrollOffset: integer }
- Set an offset for the onTotalScroll callback option.
For example, settingonTotalScrollOffset: 100
will trigger the onTotalScroll callback 100 pixels before the end of scrolling is reached.
callbacks:{ onTotalScrollBackOffset: integer }
- Set an offset for the onTotalScrollBack callback option.
For example, settingonTotalScrollBackOffset: 100
will trigger the onTotalScrollBack callback 100 pixels before the beginning of scrolling is reached.
callbacks:{ whileScrolling: function(){} }
- A function/custom code to execute while scrolling is active (callbacks demo).
Example:
callbacks:{ whileScrolling:function(){ console.log("scrolling..."); } }
callbacks:{ alwaysTriggerOffsets: boolean }
- Control the way onTotalScroll and onTotalScrollBack offsets execute.
By default, callback offsets will trigger repeatedly while content is scrolling within the offsets.
SetalwaysTriggerOffsets: false
when you need to trigger onTotalScroll and onTotalScrollBack callbacks only once.
live: boolean, "string"
- Enable or disable the creation of scroller(s) on all elements matching the current selector, now and in the future.
Setlive: true
when you need to add scroller(s) on elements that do not yet exist in the page. These could be elements added by other scripts or plugins after some action by the user.
If you need at any time to disable or enable the live option, setlive: "off"
and"on"
respectively.
You can also tell the script to disable live option after the first invocation by settinglive: "once"
.
liveSelector: "string"
- Set the matching set of elements to apply a scroller, now and in the future.
Plugin methods
Methods are ways to execute various scroller actions programmatically from within your script(s).
update
Usage $(selector).mThumbnailScroller("update");
Call the update method to manually update existing scrollers to accomodate new content or resized element(s). This method is by default called automatically by the script (via updateOnContentResize
option) when the element itself and/or its content size changes.
scrollTo
Usage $(selector).mThumbnailScroller("scrollTo", position, {options});
Call the scrollTo method to scroll content to the position parameter. Position parameter can be a string (e.g. "#element-id"
, "bottom"
, "left"
etc.), an integer indicating number of pixels (e.g. 100
), an array for y/x axis (e.g. [100,100]
), a js/jQuery object, a function etc. method demo
scrollTo position parameter
Position parameter can be:
"string"
- Element selector
For example, scroll to element with id “element-id”:
$(selector).mThumbnailScroller("scrollTo","#element-id");
- Special pre-defined position
For example, scroll to right:
$(selector).mThumbnailScroller("scrollTo","right");
Pre-defined position strings:
"bottom"
– scroll to bottom"top"
– scroll to top"right"
– scroll to right"left"
– scroll to left"first"
– scroll to the position of the first element within content"last"
– scroll to the position of the last element within content
- Number of pixels less/more: (e.g.
"-=100"
/"+=100"
)
For example, scroll by 100 pixels right:
$(selector).mThumbnailScroller("scrollTo","-=100");
- Element selector
integer
- Number of pixels (from left and/or top)
For example, scroll to 100 pixels:
$(selector).mThumbnailScroller("scrollTo",100);
- Number of pixels (from left and/or top)
[array]
- Different y/x position
For example, scroll to 100 pixels from top and 50 pixels from left:
$(selector).mThumbnailScroller("scrollTo",[100,50]);
- Different y/x position
object/function
- jQuery object
For example, scroll to element with id “element-id”:
$(selector).mThumbnailScroller("scrollTo",$("#element-id"));
- js object
For example, scroll to element with id “element-id”:
$(selector).mThumbnailScroller("scrollTo",document.getelementbyid("element-id"));
- function
For example, scroll to 100 pixels:
$(selector).mThumbnailScroller("scrollTo",function(){ return 100 });
- jQuery object
scrollTo method options
speed: integer
- Scrolling speed.
Example:
$(selector).mThumbnailScroller("scrollTo", "-=200", { speed: 30 });
duration: integer
- Scrolling animation duration, value in milliseconds.
Example:
$(selector).mThumbnailScroller("scrollTo", "right", { duration: 1000 });
easing: "string"
- Scrolling animation easing, values:
"easeInOut"
"easeOut"
"easeInOutSmooth"
"easeOutSmooth"
"easeInOutStrong"
"easeOutStrong"
"easeInOut"
"easeInOut"
Example:
$(selector).mThumbnailScroller("scrollTo", 300, { easing: "easeOutSmooth" });
callbacks: boolean
- Trigger user defined callbacks after scroll-to event is completed, values:
true
,false
.
Example:
$(selector).mThumbnailScroller("scrollTo", "top", { callbacks: false });
timeout: integer
- Method timeout (delay). The default timeout is 60 (milliseconds) in order to work with automatic scroller update functionality, value in milliseconds.
Example:
$(selector).mThumbnailScroller("scrollTo", "last", { timeout: 0 //no timeout });
stop
Usage $(selector).mThumbnailScroller("stop");
Stops running scrolling animations. Usefull when you wish to interrupt a previously scrollTo call.
disable
Usage $(selector).mThumbnailScroller("disable");
Calling disable method will temporarily disable the scroller(s). Disabled scrollers can be re-enabled by calling the update method.
To disable a scroller and reset its content position, call the method by setting its reset parameter to true
:
$(selector).mThumbnailScroller("disable", true);
destroy
Usage $(selector).mThumbnailScroller("destroy");
Calling destroy method will completely remove the scroller and return the element to its original state.
Scroller styling & themes
You can style your scroller(s) using jquery.mThumbnailScroller.css which contains the basic/default styling and few ready-to-use themes.
You can modify the default styling or an existing scroller theme directly, clone a theme and change it to your needs or overwrite the CSS in another stylesheet. If you wish to maintain compatibility with future plugin versions (that may include an updated version of jquery.mThumbnailScroller.css), I recommend using your own custom (or cloned) theme (creating your own custom theme), or overwriting the CSS. This way you can always upgrade jquery.mThumbnailScroller.css without having to redo your changes.
Themes
Plugin’s CSS includes a number of ready-to-use themes for quickly applying a basic styling to your scroller(s). To apply a theme, use theme
option parameter:
$(selector).mThumbnailScroller({ theme: "theme-name" });
or the HTML data attribute data-mts-theme
in your markup:
<div class="mThumbnailScroller" data-mts-theme="theme-name">...</div>
creating your own custom theme
User-defined callbacks
You can trigger your own js function(s) by using plugin’s callbacks option parameter (see all availbale callbacks in configuration section). Some examples:
$(selector).mThumbnailScroller({ callbacks:{ onScroll: function(){ /* do something */ } } });
$(selector).mThumbnailScroller({ callbacks:{ onTotalScroll: function(){ myFunction(); } } }); function myFunction(){ /* do something */ }
The plugin returns a number of values and objects that can be used inside the callbacks
this
– the scroller elementthis.mts.content
– the scrollable element containing the content (e.g. theul
element) as jquery objectthis.mts.top
– content’s top position (pixels)this.mts.left
– content’s left position (pixels)this.mts.topPct
– content vertical scrolling percentagethis.mts.leftPct
– content horizontal scrolling percentagethis.mts.direction
– content’s scrolling direction (returns"x"
or"y"
)
Plugin dependencies & requirements
- jQuery version 1.6.0 or higher
License
This work is released under the MIT License.
You are free to use, study, improve and modify it wherever and however you like.
https://opensource.org/licenses/MIT
Donating helps greatly in developing and updating free software and running this blog 🙂
Hello all, brother, I have a problem, how do I get when I move the scroller, to reload the page remains in the stop position, that is, if I position myself in the last image, click and you doi reloading the page, how do I appear in the position of the last picture?
Hi all,
Im looking to add page indicator to this, how could I extend this to add a callback on the previous & next buttons to update a parent div???
Thanks, G
As Will asked a long time ago, I’d like to know if someone found a way to externally call the plugin to scroll to a specific element ?
Thanks !
Ok, easy one.
$(“.jTscroller”).stop().animate({‘left’: hereYouGo});
Hello all,
Can anybody tell me how to add youtube videos in this scroller? I tried to copy and paste the youtube link but nothing show up! Do I have to add a class in the CSS for video frame? Please help, I’m so new at this!!
Thankss!!
Thanks for the script 🙂
hi dear
i used this plugin and it works successfully but 2 errors …
one that ie 9 cant run javascript to set te width … i set it manualy on css
then when i load it on an iframe it cant load buttons 🙁 what can i do
ty
gud work …. keep it up
vertical scroller is not working in IE..before 10 days it was working fine.
CRAZY COOL! super glad i found this, thanks so much for putting this together. I have been searching all over the web for something like this, most other jquery scrolls are no where near as slick as this one – keep up the great work!
Is it possible to make scroll without mouse interaction, i want to scroll the thumbnails continuously
also once the page has rendered and scroller activated. if you remove or add more images can you rerun the scroller without having to reload the page?
Hi,
2 Questions:
1st:
The hover scroll wont work on touch devices?
2nd:
I am trying to use this solution with next prev button but in vain. I am generating my images dynamically in a loop and then want to use next prev to let users browse through it. It works with hover accelerator option, but not next prev button. Do you have an example of dynamically generated images with this functionality?
thanks,
Shez
Hi,
first of all: great plugin! thanks a lot!
but I have also a question: I’m using the clickButtons and the Next button is always displayed, even when there is only one image. When you then klick the Button the single image slides to the right end. I dont like this, is i possible to disable the scroller when the content is not longer than the visible one?
hi,
figured my problem out on my own.
if anyone is insterested: it was an horizontal scroller with images of 60 px height and I set the height of the scroller also on 60 px, but since the scroller got 64 px in height (didn’f find out where the extra pixels came from til now) the scroller recognized there is content to be scrolled. since it was configured as horizontal it set the scroll buttons for left/right scrolling visible even that the content that was bigger than the bigger than the visible area was in vertical direction.
Firstly, this is a great piece of code- I really needed a vertical scroller for thumbnails and this fits the bill all ends up. It looks absolutely great in real browsers, but when I got the website I was working on up and running the vertical scroller wasn’t working in IE8 (why do I continue to be surprised by this). I then noticed that the demo 2 on this page also didn’t work in IE8 and there were several comments about IE issues. The images do not wrap in the div and the scrolling happens horizontally, the whole string of images then scrolls up and down the vertical container which is one image wide- no good at all. After much trial and error the solution turned out to be blindingly simple-
In the CSS file ‘.jThumbnailScroller .jTscrollerContainer’ is set to ‘position:absolute;’. Change it to relative and the whole thing works fine (though a background image in the container has dissappeared which is a small price to pay for the whole scroller not working- if anyone has a fix for that I would be greatful).
Malihu, thanks for the great code and if you are still monitoring this thread you might consider changing the down load file as it doesn’t appear to have had any negative effect on any other browser.
Hi, really great script but I don’t find where to change the size of the thumbnail on the verctical full page. Hope you’ll see my post really need some held here . Thanks!
Hi,
linked to my website does the scroller jQuery plugin in Opera not be cut off when scrolling images. I can not find the error. Can help me?
Thanks in advance.
Greetings Joe
Hi,
Thanks for this really wonderful plugin.
I had an issue though.
My container is full size (horizontal, 100%) and I’m using clickScrolling. Sometimes, the number of items inside the container does not exceed the container’s width, then too the images are scrollable. I want to avoid this scenario, i.e. images inside the container should be scrollable only if the number of images exceed the width of container otherwise not.
Any help regarding this would really be appreciated.
Thanks in advance.
Hello.
When i add you plugin, not work my slide show on page. When i delete your plugin from page, slide show work. Сonflicts
I added:
jQuery.noConflict();
(function ($) {
window.onload = function () {
$(“#tS2”).thumbnailScroller({
scrollerType: “hoverPrecise”,
scrollerOrientation: “horizontal”,
scrollSpeed: 2,
scrollEasing: “easeOutCirc”,
scrollEasingAmount: 600,
acceleration: 4,
scrollSpeed: 800,
noScrollCenterSpace: 10,
autoScrolling: 0,
autoScrollingSpeed: 2000,
autoScrollingEasing: “easeInOutQuad”,
autoScrollingDelay: 500
});
}
})(jQuery);
But nothing has changed. How solve this problem? Thanks for advance)
Ivo, great script! What options do I need to select if I want to create a custom build using the latest JqueryUI 1.8.18
http://jqueryui.com/download
Also, what would be necessary to add touch support, so a user could swipe the thumbnails?
Thank you so much. Nice collection
just change the class
.jThumbnailScroller#tS3 .jTscroller{height:auto; margin-bottom:0px;}
to
.jThumbnailScroller#tS3 .jTscroller{margin-bottom:0px;}
simple my friends =]
when tried to use autoscroll i get:
—
[10:44:53.832] $scroller.delay is not a function @ jquery.thumbnailScroller.js:114
from latest firefox web console – any ideas ?
I am really enjoying your script.
I have been playing with the js for a bit now, and I was wondering if maybe you had created a single image move per arrow click? Or if you haven’t, maybe a suggestion of how I would?
Thanks
Hi!
Thanks for this awesome plugin!
I have an issue regarding browser resizing though.
My container is full size (horizontal, 100%) and I’m using clickScrolling. Sometimes, the number of items inside the container does not exceed the container’s width, and when I resize the browser, the plugin “doesn’t work” (prev/next buttons are hidden).
How do I fix this? I was thinking of binding a browser resize event and
reinitialize the plugin, but it’s not working (possibly conflicting with
the previously called instance of the plugin).
And so, how can I re-bind or reinitialize the plugin?
Or possibly, can you provide me with a
destroy method for it so I can use that for the browser resize event and
I’ll just call the plugin again after (not sure though if this will work).
Hope you or anyone out there can help me with this.
Thanks again!
Did you ever get an answer to this? or come up with a solution?
I’m re-loading thumbnails with Ajax and need to reinitialize the container to re-size the scroll area. Any Ideas?
i’ve run into a similar problem as some folks above. in IE (using version 8), when the horizontal scroller in hoverAccelerate mode, the scroller bar reaches the far-right, and it seems to keep trying to scroll further, though the element is at the end, so it just kind of sits there jittering as long as the mouse is over it. if i bring my mouse back toward the middle of the scroller, the jittering becomes less intense.
any solution for this? everything else seems to work great. i’ve tweaked the CSS to fit my needs and it looks great. i just can’t have it skipping like a stone when i get to the end of the ribbon!
thanks 🙂
i actually figured this out just now. basically the script for the hoverAccelerate was referring to the element’s left for both sides, instead of “left” and then “right”, so when the ribbon reached the far right, it was doing some math based on the left position. does that make sense?
this is what WAS in the JavaScript:
function Scrolling(){ if((mouseCoords=0)){ $scroller.stop(true,true).css("left",0); }else if((mouseCoords>$this.width()/2) && ($scroller.position().left<=-(totalWidth-$this.width()))){ $scroller.stop(true,true).css("left",-(totalWidth-$this.width())); }else{ if((mouseCoords =($this.width()/2)+options.noScrollCenterSpace)){ scrollerPos=Math.round(Math.cos((mouseCoords/$this.width())*Math.PI)*(interval+options.acceleration)); $scroller.stop(true,true).animate({left:"+="+scrollerPos},interval,"linear"); }else{ $scroller.stop(true,true); } }
and i tweaked it to be this:
function Scrolling(){ if((mouseCoords=0)){ $scroller.stop(true,true).css("left",0); }else if((mouseCoords>$this.width()/2) && ($scroller.position().left<=-(totalWidth-$this.width()))){ $scroller.stop(true,true).css("right",-(totalWidth-$this.width())); }else{ if((mouseCoords =($this.width()/2)+options.noScrollCenterSpace)){ scrollerPos=Math.round(Math.cos((mouseCoords/$this.width())*Math.PI)*(interval+options.acceleration)); $scroller.stop(true,true).animate({left:"+="+scrollerPos},interval,"linear"); }else{ $scroller.stop(true,true); } }
had same problem – since autoscrolling is not working i turned autoScrolling:0 in options and it is ok now
Can we reach to specific image in horizontal scroller using javasript. e.g I am on first image and on click of any button, scroller should take me to 8th image?
Hello friend! It would be possible to activate simultaneously clickButtons and hoverAccelerate? I think this way could solve the problem of navigation with iPad. Do you agree? Thanks and good luck.
Is there any way to make prettyPhoto work with it ?
Currently i placed in my site older version of this horizontal scroller but it scrolls crazy fast and there’s no parameter of scrollspeed, only “mouseover fade speed”. Maybe in older version there is some trick to slow this mouse over scrolling ?
This is awesome. So simple and so easy to use. Looks awesome. Thanks!!
Thanks !
I have loads of pictures and have been looking for a lovely easy way to share them online so they look professional too !