jQuery thumbnail scroller
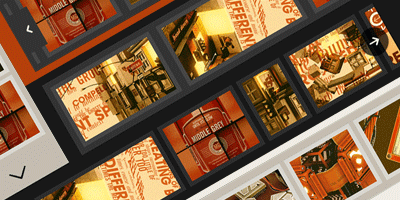
A thumbnail/image scroller that can be used as standalone or alongside lightboxes, gallery scripts etc. Features include: scrolling by cursor movement, buttons and/or touch, vertical and/or horizontal scrolling, customization via CSS and option parameters, methods for triggering events like scroll-to, update, destroy etc., user-defined callbacks functions and more.
Current version 2.0.3 (Changelog) – Version 1.x.x (no longer actively maintained)
How to use it
HTML
Get plugin files. Extract and upload jquery.mThumbnailScroller.min.js and jquery.mThumbnailScroller.css to your web server (more info)
Include jquery.mThumbnailScroller.css in the head tag your html document
<link rel="stylesheet" href="/path/to/jquery.mThumbnailScroller.css">
Include jQuery library (if your project doesn’t use it already) and jquery.mThumbnailScroller.min.js in your document’s head or body tag
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script> <script src="/path/to/jquery.mThumbnailScroller.min.js"></script>
Create the markup with your images, links, etc. which is basically an element (e.g. a div) holding an unordered list (ul)
<div id="my-thumbs-list"> <ul> <li><a href="image-1-link"><img src="/path/to/image-1-file" /></a></li> <li><a href="image-2-link"><img src="/path/to/image-2-file" /></a></li> <li><a href="image-3-link"><img src="/path/to/image-3-file" /></a></li> <li><a href="image-4-link"><img src="/path/to/image-4-file" /></a></li> <!-- and so on... --> </ul> </div>
CSS
Give your element (in the example above #my-thumbs-list) a width and/or height value and a CSS overflow value of auto or hidden. For example:
#my-thumbs-list{ overflow: auto; width: 800px; height: auto; }
If you’re creating a vertical scroller, you’ll need to set a height (or max-height) value
#my-thumbs-list{ overflow: auto; width: 300px; height: 500px; }
Initialization
Initialize via javascript
Create a script and call mThumbnailScroller function on your element selector along with the option parameters you want
<script> (function($){ $(window).load(function(){ $("#my-thumbs-list").mThumbnailScroller({ axis:"x" //change to "y" for vertical scroller }); }); })(jQuery); </script>
Initialize via HTML
If you prefer to initialize the plugin without a script, you can simply give your element the class mThumbnailScroller
and optionally set its orientation via the HTML data attribute data-mts-axis
("x"
for horizontal and "y"
for vertical). For example:
<div id="my-thumbs-list" class="mThumbnailScroller" data-mts-axis="x"> <ul> <!-- your content --> </ul> </div>
Basic configuration & option parameters
The 2 basic options are axis
which defines the scroller orientation and type
which defines how the user interacts with the scroller.
axis
The default scroller orientation is horizontal, so if you don’t set an axis
option value, it defaults to "x"
$("#my-thumbs-list").mThumbnailScroller({ axis:"x" });
To create a vertical scroller, set axis
option to "y"
$("#my-thumbs-list").mThumbnailScroller({ axis:"y" });
or use data-mts-axis="y"
if you’re initializing the plugin via HTML
type
You can set the type of scroller via javascript, for example:
$("#my-thumbs-list").mThumbnailScroller({ type:"hover-50" });
or using the HTML data attribute data-mts-type
, e.g.:
<div class="mThumbnailScroller" data-mts-type="hover-50">...</div>
There are 4 basic scroller types:
"hover-[number]"
(e.g."hover-33"
,"hover-80"
etc.)Scrolling content in linear mode by hovering the cursor over the scroller edges. The edges are defined by the type name, where [number] indicates the percentage of the scroller area in which scrolling is idle. For example, setting
"hover-50"
(default value) on a 600 pixels wide horizontal scroller, means that scrolling will be triggered only when the cursor is over the first 150 pixels from the left or right edge. And when cursor is over the 50% of the scroller width (300 pixels), scrolling will be idle or stopped.hover-precise
Scrolling content with animation easing (non-linear mode) by hovering the cursor over the scroller. The scrolling speed and direction is directly affected by the cursor movement and position within the scroller.
"click-[number]"
(e.g."click-50"
,"click-90"
etc.)Scrolling content by clicking buttons. The scrolling amount is defined by type name, where [number] indicates a percentage of the scroller area. For example, setting
"click-50"
on a 800 pixels wide horizontal scroller, means that each time you click the left or right arrow buttons, the content will scroll by 400 pixels (50% of 800px) to the left or right.click-thumb
Scrolling each image/thumbnail at a time by clicking buttons.
"hover-[number]"
and hover-precise
types become disabled on touch-enabled devices and replaced by content touch-swipe scrolling.
Configuration
You can configure your scroller(s) using the following option parameters when calling mThumbnailScroller function
Usage $(selector).mThumbnailScroller({ option: value });
setWidth: false
- Set the width of the scroller (overwrites CSS width), value in pixels (integer) or percentage (string).
setHeight: false
- Set the height of the scroller (overwrites CSS height), value in pixels (integer) or percentage (string).
setTop: 0
- Set the initial css top property of content, accepts string values (css top position).
Example:setTop: "-100px"
.
setLeft: 0
- Set the initial css left property of content, accepts string values (css left position).
Example:setLeft: "-100px"
.
type: "string"
- Scroller type defines the way users interact with the scroller.
Available values:"hover-[number]"
(e.g."hover-50"
)
Scrolling content in linear mode by hovering the cursor over the scroller edges. The edges are defined by the type name, where [number] indicates the percentage of the scroller area in which scrolling is idle. Scrolling accelerates proportionally according to cursor position: e.g. scrolling speed increases as the cursor moves towards the edges and decreases as it moves towards the center of the scroller area."hover-precise"
Scrolling content with animation easing (non-linear mode) by hovering the cursor over the scroller. The scrolling speed and direction is directly affected by the cursor movement and position within the scroller.click-[number]
(e.g."click-80"
)
Scrolling content by clicking buttons. The scrolling amount is defined by type name, where [number] indicates a percentage of the scroller area."click-thumb"
Scrolling each image/thumbnail at a time by clicking buttons. Each click will scroll the content by the width/height of the next non-visible adjusted image.
axis: "string"
- Define scroller axis (scrolling orientation).
Available values:"y"
,"x"
,"yx"
.axis: "y"
– vertical scrolleraxis: "x"
– horizontal scroller (default)axis: "yx"
– vertical and horizontal scroller (useful when panning images or switching axis on-the-fly)
speed: integer
- Set the scrolling speed (higher=faster). Default value is
15
.
contentTouchScroll: integer
- Enable or disable content touch-swipe scrolling for touch-enabled devices.
To completely disable, setcontentTouchScroll: false
. Integer values define the axis-specific minimum amount required for scrolling momentum (default value is25
).
markup:{ thumbnailsContainer: "string" }
- Set the element containing your thumbnails. By default, this is an unordered list (
ul
).
markup:{ thumbnailContainer: "string" }
- Set the element containing each thumbnail. By default this is a list-item (
li
).
markup:{ thumbnailElement: "string" }
- Set the actual thumbnail element. By default, this is an image tag (
img
).
advanced:{ autoExpandHorizontalScroll: boolean }
- Auto-expand content horizontally (for
"x"
or"yx"
axis).
If set totrue
(default), content will expand horizontally to accomodate any floated/inline-block elements (such asli
,a
,img
etc.).
advanced:{ updateOnContentResize: boolean }
- Update scroller(s) automatically on content, element or viewport resize. The value should be
true
(default) for fluid layouts/elements, adding/removing content dynamically, hiding/showing elements etc.
advanced:{ updateOnImageLoad: boolean }
- Update scroller(s) automatically each time an image inside the element is fully loaded. The value is
true
by default.
advanced:{ updateOnSelectorChange: "string" }
- Update scroller(s) automatically when the amount and size of specific selectors changes.
Useful when you need to update the scroller(s) automatically, each time a type of element is added, removed or changes its size.
For example, settingupdateOnSelectorChange: "ul li"
will update the scroller each time list-items inside the element are changed. Setting the value totrue
, will update the scroller each time any element is changed. To disable (default) set tofalse
.
theme: "string"
- Set a ready-to-use scroller theme (plugin’s CSS contains all themes).
callbacks:{ onInit: function(){} }
- A function/custom code to execute when the scroller has initialized (callbacks demo).
Example:
callbacks:{ onInit:function(){ console.log("scroller initialized"); } }
callbacks:{ onScrollStart: function(){} }
- A function/custom code to execute when scrolling starts (callbacks demo).
Example:
callbacks:{ onScrollStart:function(){ console.log("scroll started"); } }
callbacks:{ onScroll: function(){} }
- A function/custom code to execute when scrolling is completed (callbacks demo).
Example:
callbacks:{ onScroll:function(){ console.log("scroll completed"); } }
callbacks:{ onTotalScroll: function(){} }
- A function/custom code to execute when scrolling is completed and content is scrolled all the way to the end (bottom and/or right) (callbacks demo).
Example:
callbacks:{ onTotalScroll:function(){ console.log("Scrolled to 100%"); } }
callbacks:{ onTotalScrollBack: function(){} }
- A function/custom code to execute when scrolling is completed and content is scrolled back to the beginning (top and/or left) (callbacks demo).
Example:
callbacks:{ onTotalScrollBack:function(){ console.log("Scrolled back to 0%"); } }
callbacks:{ onTotalScrollOffset: integer }
- Set an offset for the onTotalScroll callback option.
For example, settingonTotalScrollOffset: 100
will trigger the onTotalScroll callback 100 pixels before the end of scrolling is reached.
callbacks:{ onTotalScrollBackOffset: integer }
- Set an offset for the onTotalScrollBack callback option.
For example, settingonTotalScrollBackOffset: 100
will trigger the onTotalScrollBack callback 100 pixels before the beginning of scrolling is reached.
callbacks:{ whileScrolling: function(){} }
- A function/custom code to execute while scrolling is active (callbacks demo).
Example:
callbacks:{ whileScrolling:function(){ console.log("scrolling..."); } }
callbacks:{ alwaysTriggerOffsets: boolean }
- Control the way onTotalScroll and onTotalScrollBack offsets execute.
By default, callback offsets will trigger repeatedly while content is scrolling within the offsets.
SetalwaysTriggerOffsets: false
when you need to trigger onTotalScroll and onTotalScrollBack callbacks only once.
live: boolean, "string"
- Enable or disable the creation of scroller(s) on all elements matching the current selector, now and in the future.
Setlive: true
when you need to add scroller(s) on elements that do not yet exist in the page. These could be elements added by other scripts or plugins after some action by the user.
If you need at any time to disable or enable the live option, setlive: "off"
and"on"
respectively.
You can also tell the script to disable live option after the first invocation by settinglive: "once"
.
liveSelector: "string"
- Set the matching set of elements to apply a scroller, now and in the future.
Plugin methods
Methods are ways to execute various scroller actions programmatically from within your script(s).
update
Usage $(selector).mThumbnailScroller("update");
Call the update method to manually update existing scrollers to accomodate new content or resized element(s). This method is by default called automatically by the script (via updateOnContentResize
option) when the element itself and/or its content size changes.
scrollTo
Usage $(selector).mThumbnailScroller("scrollTo", position, {options});
Call the scrollTo method to scroll content to the position parameter. Position parameter can be a string (e.g. "#element-id"
, "bottom"
, "left"
etc.), an integer indicating number of pixels (e.g. 100
), an array for y/x axis (e.g. [100,100]
), a js/jQuery object, a function etc. method demo
scrollTo position parameter
Position parameter can be:
"string"
- Element selector
For example, scroll to element with id “element-id”:
$(selector).mThumbnailScroller("scrollTo","#element-id");
- Special pre-defined position
For example, scroll to right:
$(selector).mThumbnailScroller("scrollTo","right");
Pre-defined position strings:
"bottom"
– scroll to bottom"top"
– scroll to top"right"
– scroll to right"left"
– scroll to left"first"
– scroll to the position of the first element within content"last"
– scroll to the position of the last element within content
- Number of pixels less/more: (e.g.
"-=100"
/"+=100"
)
For example, scroll by 100 pixels right:
$(selector).mThumbnailScroller("scrollTo","-=100");
- Element selector
integer
- Number of pixels (from left and/or top)
For example, scroll to 100 pixels:
$(selector).mThumbnailScroller("scrollTo",100);
- Number of pixels (from left and/or top)
[array]
- Different y/x position
For example, scroll to 100 pixels from top and 50 pixels from left:
$(selector).mThumbnailScroller("scrollTo",[100,50]);
- Different y/x position
object/function
- jQuery object
For example, scroll to element with id “element-id”:
$(selector).mThumbnailScroller("scrollTo",$("#element-id"));
- js object
For example, scroll to element with id “element-id”:
$(selector).mThumbnailScroller("scrollTo",document.getelementbyid("element-id"));
- function
For example, scroll to 100 pixels:
$(selector).mThumbnailScroller("scrollTo",function(){ return 100 });
- jQuery object
scrollTo method options
speed: integer
- Scrolling speed.
Example:
$(selector).mThumbnailScroller("scrollTo", "-=200", { speed: 30 });
duration: integer
- Scrolling animation duration, value in milliseconds.
Example:
$(selector).mThumbnailScroller("scrollTo", "right", { duration: 1000 });
easing: "string"
- Scrolling animation easing, values:
"easeInOut"
"easeOut"
"easeInOutSmooth"
"easeOutSmooth"
"easeInOutStrong"
"easeOutStrong"
"easeInOut"
"easeInOut"
Example:
$(selector).mThumbnailScroller("scrollTo", 300, { easing: "easeOutSmooth" });
callbacks: boolean
- Trigger user defined callbacks after scroll-to event is completed, values:
true
,false
.
Example:
$(selector).mThumbnailScroller("scrollTo", "top", { callbacks: false });
timeout: integer
- Method timeout (delay). The default timeout is 60 (milliseconds) in order to work with automatic scroller update functionality, value in milliseconds.
Example:
$(selector).mThumbnailScroller("scrollTo", "last", { timeout: 0 //no timeout });
stop
Usage $(selector).mThumbnailScroller("stop");
Stops running scrolling animations. Usefull when you wish to interrupt a previously scrollTo call.
disable
Usage $(selector).mThumbnailScroller("disable");
Calling disable method will temporarily disable the scroller(s). Disabled scrollers can be re-enabled by calling the update method.
To disable a scroller and reset its content position, call the method by setting its reset parameter to true
:
$(selector).mThumbnailScroller("disable", true);
destroy
Usage $(selector).mThumbnailScroller("destroy");
Calling destroy method will completely remove the scroller and return the element to its original state.
Scroller styling & themes
You can style your scroller(s) using jquery.mThumbnailScroller.css which contains the basic/default styling and few ready-to-use themes.
You can modify the default styling or an existing scroller theme directly, clone a theme and change it to your needs or overwrite the CSS in another stylesheet. If you wish to maintain compatibility with future plugin versions (that may include an updated version of jquery.mThumbnailScroller.css), I recommend using your own custom (or cloned) theme (creating your own custom theme), or overwriting the CSS. This way you can always upgrade jquery.mThumbnailScroller.css without having to redo your changes.
Themes
Plugin’s CSS includes a number of ready-to-use themes for quickly applying a basic styling to your scroller(s). To apply a theme, use theme
option parameter:
$(selector).mThumbnailScroller({ theme: "theme-name" });
or the HTML data attribute data-mts-theme
in your markup:
<div class="mThumbnailScroller" data-mts-theme="theme-name">...</div>
creating your own custom theme
User-defined callbacks
You can trigger your own js function(s) by using plugin’s callbacks option parameter (see all availbale callbacks in configuration section). Some examples:
$(selector).mThumbnailScroller({ callbacks:{ onScroll: function(){ /* do something */ } } });
$(selector).mThumbnailScroller({ callbacks:{ onTotalScroll: function(){ myFunction(); } } }); function myFunction(){ /* do something */ }
The plugin returns a number of values and objects that can be used inside the callbacks
this
– the scroller elementthis.mts.content
– the scrollable element containing the content (e.g. theul
element) as jquery objectthis.mts.top
– content’s top position (pixels)this.mts.left
– content’s left position (pixels)this.mts.topPct
– content vertical scrolling percentagethis.mts.leftPct
– content horizontal scrolling percentagethis.mts.direction
– content’s scrolling direction (returns"x"
or"y"
)
Plugin dependencies & requirements
- jQuery version 1.6.0 or higher
License
This work is released under the MIT License.
You are free to use, study, improve and modify it wherever and however you like.
https://opensource.org/licenses/MIT
Donating helps greatly in developing and updating free software and running this blog 🙂
Great code!
Hello,
there seems to be a problem with jQuery v3.6.0
When page is loaded, I get e.typeof is undefined.
The workaround that was to install the following:
Is there any plans to upgrade the jQuery thumbnail scroller to fully support jQuery v3.6.0 and above ?
hi
i was searching a vertical scroller and got your code. thanks a lot.
i have one question. how can i show content/text on right side when i click on the thumbnail. is it possible?
Hi, Nice & Informative article. Web designing company in Hyderabad is one of the best practices nowadays most of the organizations from startup to big brands are using. It’s very helpful in showcasing our business to the right person in right time. Very nice article. Found informative for me.
Hi… Thank you so much for your plugin. You have no idea how you’ve saved me. It works great. But I was wondering… is it possible to also make the thumbnails scroll by using the mouse-wheel, that is, in addition to the hovering over the edges??
Kind regards,
Carla.
Hey Guys!
Any idea how to implement this in a Shopify store? I know I have to add the jv and css files in the assets but what should I do next?
Please help
Why I can’t load data if my data more than 21 data from database ?
Trying to figure out how to change the scroll speed. Integer and ‘scrollSpeed’ do not work for me. Any ideas? I am changing it in jquery.thumbnailScroller.js file. Thanks in advance.
It’s 2018 btw, not sure what changes have been made in the last couple years to warrant any code not working.
how can do that slider will auto-scroll
Harsh Prajapati
Can we stop scrolling on mouse click or hover wherever we need.
Hi,
Great plugin,Working perfectley ,
Is it possible to add multi[le scrolling methord for a single id.
like both click and hover
$(“#my-thumbs-list”).mThumbnailScroller({
axis:”y” ,//change to “y” for vertical scroller,
type:”hover-50″,
contentTouchScroll :50
});
$(“#my-thumbs-list”).mThumbnailScroller({
axis:”y” ,//change to “y” for vertical scroller,
type:”click-50″,
});
.mTS_horizontal .mTSContainer { margin-left: auto !important; margin-right: auto !important; }
Malihu, thank you for such a great js plugin!
I have only a small issue: can’t center thumbnails if their overall width is less than of the container. So they are aligned to the left.
If I’m not blind (don’t see in config section) and such feature is not implemented in this version of the plugin, I’m requesting such feature in new releases! 🙂
Yes, this can be achived with callbacks-counting-offset, but it becomes complicated.
What do You think, Malihu?
Old comment I know, but wanted the same thing. Simply css your img to be display:block and margin:auto.
img {
display: block;
margin: auto;
}
hi Thanks for this great Job!
I want to scroll a grid box of 15 list items and display 9 list items first and 6 list item… when scroll is activated.
Can u help me please
EXcellent posts..
sap netweaver training in hyderabad
Hi this is a great script for me. please could you explain how I can get this to work on desktop using hover and same time on Mobile using touch
The plugin works with regular mouse and touch (you don’t have to do anything). In touch devices you just touch swipe thumbnails. Do you have a particular issue with a device?
The scroller goes on infinite scroll on touch devices. It won’t stop at the end of the list but keep on scrolling white no any elements inside, just white background.
Hi!
Great plugin!
Have a question about optimalization. When I use a multilevel thumbnails grid to move entire website from left to right there is a problem with smothness. There’s little of shaking. When I use 32gb ram, i7 pc its works fine but in regular pc’s there is a problem with that. Have any suggestion?:)
Regards,
Alek
If wanted to initially scroll to the middle of thumbnail (or other) content, how would i do that? element.scrollLeft(n) doesnt seem to work and seems to be overwritten?
My div is 5000 px wide and i want to initially show it @ 2500 px.
Hi, thanks for this great script.
But I have a question: Is it possible, that the images are continuing scrolling while holding down the mouse button over the arrows instead of clicking the button?
Hi,
Using mousedown/up events can be a bit tricky with touch devices but I have a working example here:
http://manos.malihu.gr/repository/jquery-thumbnail-scroller/demo/examples/mousedown-buttons-test.html
View the source and adapt the code to your needs. The code that does the trick is the last script tag (right before the closing body tag). Hope this helps 🙂
Hi,
how to turn on infinitve scrolling?
Hi,
Infinite scrolling is not part of plugin’s core so there’s no single option to enable it automatically.
You can do it using few lines of extra code within the callback options. See the working example below:
http://manos.malihu.gr/repository/jquery-thumbnail-scroller/tests/examples/carousel-test.html
Note that such functionality will only work with scroller buttons and not with hover.
Excellent plugin. It works well. I used it to implement horizontal scrolling playlist for JWPlayer 7. See screenshot: http://pakjiddat.com/wp-content/uploads/2016/02/jwplayer-integration-750×410.png.
Thanks for your plugin.
Regards,
Nadir Latif