Simple navigation with CSS3 and jQuery
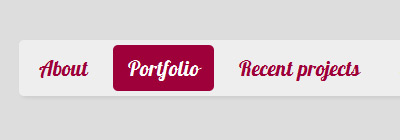
A simple navigation menu built with CSS3 and the jQuery UI.
view demo Additional demo with selected state
download Get all files related to post
The css with some custom fonts via Google font API
@import url(http://fonts.googleapis.com/css?family=Lobster); body {margin:0; padding:0; background:#ddd;} #nav{position:relative; margin:40px; background:#eee; padding:0; font-family:'Lobster', Arial, Helvetica, sans-serif; font-size:21px; -moz-border-radius:5px; -khtml-border-radius:5px; -webkit-border-radius:5px; border-radius:5px; -moz-box-shadow:2px 2px 3px #ccc; -webkit-box-shadow:2px 2px 3px #ccc; box-shadow:2px 2px 3px #ccc;} #nav .clear{clear:both;} #nav ul{padding:0 0 0 5px; margin:0; list-style:none;} #nav li{float:left; margin:5px 10px 5px 0; background:#eee; -moz-border-radius:5px; -khtml-border-radius:5px; -webkit-border-radius:5px; border-radius:5px;} #nav li a{text-decoration:none; color:#9e0039; display:block; padding:10px 15px;}
Load the jquery library and UI straight from Google
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.4/jquery.min.js" type="text/javascript"></script> <script src="http://ajax.googleapis.com/ajax/libs/jqueryui/1.8/jquery-ui.min.js" type="text/javascript"></script>
The jQuery code
<script> $(document).ready(function(){ $nav_li=$("#nav li"); $nav_li_a=$nav_li.children("a"); var animSpeed=450; //fade speed var hoverTextColor="#fff"; //text color on mouse over var hoverBackgroundColor="#9e0039"; //background color on mouse over var textColor=$nav_li_a.css("color"); var backgroundColor=$nav_li.css("background-color"); $nav_li_a.hover(function() { var $this=$(this); $this.stop().animate({ color: hoverTextColor }, animSpeed).parent().stop().animate({ backgroundColor: hoverBackgroundColor }, animSpeed); },function() { var $this=$(this); $this.stop().animate({ color: textColor }, animSpeed).parent().stop().animate({ backgroundColor: backgroundColor }, animSpeed); }); }); </script>
Optional jQuery code that features a selected state when an option is clicked
<script> $(document).ready(function(){ $nav_li=$("#nav li"); $nav_li_selected=$("#nav li.selected"); $nav_li_a=$nav_li.children("a"); var animSpeed=450; //fade speed var hoverTextColor="#fff"; //text color on mouse over var hoverBackgroundColor="#9e0039"; //background color on mouse over var textColor=$nav_li_a.css("color"); var backgroundColor=$nav_li.css("background-color"); //init selected $nav_li_selected.css("background-color",hoverBackgroundColor).children().css("color",hoverTextColor); $nav_li_a.hover(function() { var $this=$(this); $this.stop().animate({ color: hoverTextColor }, animSpeed).parent().stop().animate({ backgroundColor: hoverBackgroundColor }, animSpeed); },function() { var $this=$(this); if(!$this.parent().is(".selected")){ $this.stop().animate({ color: textColor }, animSpeed).parent().stop().animate({ backgroundColor: backgroundColor }, animSpeed); } }); //selected state $nav_li_a.click(function(){ var $this=$(this); $this.stop().animate({ color: hoverTextColor }, animSpeed).parent().stop().animate({ backgroundColor: hoverBackgroundColor }, animSpeed).addClass("selected").siblings(".selected").removeClass("selected").stop().animate({ backgroundColor: backgroundColor }, animSpeed).children().stop().animate({ color: textColor }, animSpeed); }); }); </script>
The html markup
<div id="nav"> <ul> <li><a href="#about">About</a></li> <li><a href="#portfolio">Portfolio</a></li> <li><a href="#recent">Recent projects</a></li> <li><a href="#experiments">Experiments / miscellaneous</a></li> <li><a href="#contact">Contact me</a></li> </ul> <div class="clear"></div> </div>
Optionally, if you need to have a menu option selected on page load, add the class-name selected on it. For example:
<li class="selected"><a href="#about">About</a></li>
To add support for rounded corners in IE8 we can use DD_roundies script by Drew Diller. Add the following code inside the head tags:
<script src="DD_roundies_0.0.2a-min.js"></script> <script> DD_roundies.addRule("#nav", "5px"); DD_roundies.addRule("#nav li", "5px"); </script>
Thatβs it. Feel free to modify it as you like π
Changelog
- Oct 27, 2011
- Added an optional selected state for menu options (as requested by some users)
Code is much shorter and a bit more optimized
- Added an optional selected state for menu options (as requested by some users)
como hacer un submenu?
So i am having trouble making this work with wordpress. Works on the homepage but if I go to any other page it fails to work and also never remembers the new page.
Thanks…
Oh, and any thoughts on child menu items?
Working on client site and would love the “selected” state to work but is not. Using this in WordPress so I have a dynamic menu and cannot manually place a class in the . I thought the code was making that happen dynamically, but perhaps I’m wrong. Are there special needs for using in WordPress with wp_nav_menu?
How to install to your website / blog how the heck?
Please guidance, I was the new guy on Websites & Blogs
# If you not mind, could be a senpai / my teacher?
Please add, http://fb.com/Nsx.Nsx097
Thank you.
hi ! how to highlight current menu item because with just css this leads to strange behaviour?
Hi,
See the additional demo with a selected state:
http://manos.malihu.gr/tuts/jquery_css3_simple_menu_selected_state.html
Hi! This code is a great piece of code, thanks a lot for your work! π
I’ve been trying to achieve something but I can’t do what I want, maybe you can help me: I don’t want any right margin on the last button.
I tried:
#nav > li:last-child {margin-right:0px;}
But it doesn’t work. If you have any idea how I can do that, thanks for your help!
J.
Hi and thanks for your comments π
Try without the > symbol
#nav li:last-child{margin-right:0;}
this will overwrite
#nav li{margin:5px 10px 5px 0;}
Thanks a lot malihu, I should have tried that but I thought the > symbol was the right way to put it.
It works fine on FF but not on IE8. I’ll search if there is a way to make this work with IE8.
Thanks again! π
J.
thank you so much guy!!! it so wonderful. Can it run in IE???
Nice Navigation.
Hope can do some code cleanup.
<div id="nav"> <ul> <li><a href="#about">About</a></li> <li><a href="#portfolio">Portfolio</a></li> <li><a href="#recent">Recent projects</a></li> <li><a href="#experiments">Experiments / miscellaneous</a></li> <li><a href="#contact">Contact me</a></li> </ul> <div class="clear"></div> </div>
Just by adding
$nav_li.prepend('<span> </span>');
in jQuery part?
Above comment goes to “menu with images”
Thank you for nice menu with background animation.
I love this navigation, it’s so simple and elegant. Just one problem when customising the CSS.
I removed the background colours for the UL and LI, so that I can have text links over a body background (colour, but could be graphic later). And I found that the mouse out LI background-color was always white. I couldn’t see this white referenced anywhere.
Finally found out that the #nav li {background:#???} is the colour used on mouseOut and if that isn’t specified, the browser uses white.
I’d like the background to be transparent, but setting this value in the CSS for this element doesn’t work. Can’t figure out why, it’s a valid CSS value. Must be something to do with the function.
Is it possible to have the initial and mouseOut LI colour as transparent?
Not really. You cannot animate to transparency with jQuery UI.
Pity, thanks for the clarification and thanks again for the code.
This is awesome work, keep up the good stuff!! I absolutely love this post, I’ve used it on my new site along with your image panning tutorial. Cheers π
Yeach, i works best. thanks a lot.
Hi, Its great menu , i tried to use it in my site , but when i remove the # and add the page link , for example about.html , the selected state never work .
is there idea i can use it to fix this issue please ?
Thanks
Hello Rasha,
The selected state only works for single-page navigation, e.g. when scrolling to anchor points on the same page. To apply a selected state on a navigation menu across multiple pages you’d normally have to use some server-side language such as PHP.
Can you recommend a tutorial to help me in this issue please? i just need the navigation button to be changed to another color when its active
Thanks
el menu es posible crear submenu ?
Hello,
I like this menu very much, but have one problem using it.
How do I have to change the code (maybe only the css part), that after I have clicked on one menu entry this stay in front? (I’m using php or asp, so after clicking a menu entry and reloading the site I could use a css class or something else to highlight the selected menu entry…but my css is bad so I do not find the solution…)
Done π
Hello,
is it possible that every li background is colored differently?
Hello,
Do you want to have different colors for each menu entry for hover only or for both?
Hey,
I only wanted to make different backgrounds for hover states… Every li gets it’s own background color on hover…
Thanks for fast reply!
This is very nice navigation. I really like how i made mine look!
This navigation is wonderful! We would like to use it in our template however with background images for hover state. Is it possible to give a background image URL rather than background color? Sorry we don’t know much about jQuery.
The way the script works, it animates background colors using the jquery UI. To switch two images (one image for mouse-over and one for mouse-out) you’d need to insert some additional divs (that’ll have as background image the mouse-over/out images) with different z-index and use a fadeIn-fadeOut jquery function on hover. I might be able to make a script like that on the coming weekend if you’d like.
Great! We’d appreciate if you can make such a script.
Hello again,
I’ve made the script. Check it at:
http://manos.malihu.gr/tuts/jquery_simple_menu_images.html
Have fun π
Thank you so much π
@Virtual Crowds
Of course it’s fine π Thanks!
@XHTML Valid Websites
Cool π
Check it out here:
http://xhtml-valid-websites.com/forum/Thread-full-size-background-with-jquery-navigation
Which modules of jQuery UI are used? Want to do a “smallest” version.
Just use the Color Animation – http://jqueryui.com/demos/animate/
Yeah, just playin around with it.
I’ll post a link to it if you want π
Sure π
This is a pretty nice nav menu. I have to play with it and will integrate it on one of my sites… π
Thanks for sharing this beauty.
Your welcome . You can do lots of nice stuff with it π
sir i am new to this section. sir how can i use these scripts in a html page…